
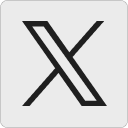




Dictionaries are a built-in data structure in Python that allows you to store and retrieve key-value pairs. You can initialize a dictionary in a few different ways, depending on what you’re trying to do.
Initialize an Empty Dictionary
The simplest way to initialize an empty dictionary in Python is to use the curly braces {}.
For example:
empty_dict = {}
This creates a new empty dictionary so you can add key-value pairs later.
Initialize a Dictionary with Values
You can also initialize a dictionary with values by providing a list of key-value pairs separated by colons.
For example:
states = {"CA": "California", "NY": "New York", "TX": "Texas"}
This creates a new dictionary called “states” that has three key-value pairs: “CA” maps to “California,” “NY” maps to “New York,” and “TX” maps to “Texas.”
You can also use the dict() constructor to initialize a dictionary with values. The dict() constructor takes an iterable (like a list or tuple) of key-value pairs and creates a new dictionary.
states = dict([("CA", "California"), ("NY", "New York"), ("TX", "Texas")])
The dict() constructor also accepts keyword arguments. In that case, the syntax becomes
states = dict(CA="California", NY="New York", TX="Texas")
Related: Python Check If Variable Is None
Initialize a Dictionary with Another Dictionary
You can also create a new dictionary by initializing it with another dictionary.
For example:
original_dict = {"CA": "California", "NY": "New York", "TX": "Texas"}
copy_dict = dict(original_dict)
This creates a new dictionary called “copy_dict” which is a copy of the “original_dict” dictionary. This is useful if you want to make a copy of a dictionary so you can modify it without changing the original.
You can also use the copy() method of a dictionary. This gives the same result as above.
copy_dict = original_dict.copy()
Initialize a Dictionary with a Comprehension
Dictionary comprehension is a concise way to create a dictionary. It consists of an expression followed by a “for” clause and zero or more “if” clauses. The expression is evaluated for each item in the “for” clause, and the resulting value is used as a key in the new dictionary. The value associated with that key is the value of the expression.
A simple example:
squared_dict = {x: x**2 for x in range(10)}
This creates a new dictionary called “squared_dict” with key-value pairs where the keys are the integers from 0 to 9, and the values are the squares of those integers.
There’s a lot you can do with dictionaries in Python, and initializing them in different ways is an important first step.
Update and Add Items to a Dictionary
Once you’ve initialized a dictionary, you may need to add or update key-value pairs. You can add a key-value pair to a dictionary by assigning a value to a new key.
For example:
states = {"CA": "California", "NY": "New York", "TX": "Texas"}
states["FL"] = "Florida"
This adds a new key-value pair of “FL” and “Florida” to the “states” dictionary.
You can update an existing value by simply reassigning it to an existing key.
states["NY"] = "New York City"
This changes the value associated with the key “NY” from “New York” to “New York City.”
Delete Items From a Dictionary
The del statement can delete a key-value pair from a dictionary.
del states["NY"]
This deletes the key “NY” and its associated value from the “states” dictionary.
You can also use the pop()
method to delete a key-value pair and return its value.
state_name = states.pop("CA")
print(state_name) # "California"
This will remove the key “CA” along with its value “California” and assign that value to state_name
Iterate Over a Dictionary
You can iterate over the keys, values, or items of a dictionary using a for
loop.
For example, to iterate over the keys of a dictionary:
for key in states:
print(key)
This will print each key in the “states” dictionary in no particular order.
To iterate over the values of a dictionary:
for value in states.values():
print(value)
To iterate over the items of a dictionary, which is a tuple of key-value pairs:
for key, value in states.items():
print(key, value)
Related: [Solved] “TypeError: ‘list’ object is not callable” in Python
Conclusion
In this article, I have covered different ways to initialize a dictionary in Python, such as using curly braces, the dict() constructor, and dictionary comprehension. I also discussed how to add, update, and delete items from a dictionary and how to iterate over the keys, values, and items of a dictionary.
Dictionaries are a powerful data structure in Python that can make your code more efficient and readable. Understanding the different ways to create and manipulate dictionaries is an important step in becoming a proficient Python programmer.
Frequently Asked Questions
What is the difference between a list and a dictionary?
A list is an ordered collection of items, whereas a dictionary is a collection of key-value pairs. Lists are indexed by integers, whereas keys index dictionaries. Lists are accessed by referencing the index number, whereas dictionaries are accessed by referencing the key.
In summary, lists are used when you want to keep things in order and access them by index, while dictionaries are used to access items by keys quickly.
Can I use a list as a key in a dictionary?
No, you cannot use a list as a key in a dictionary because lists are mutable (can be changed) and thus are not hashable (cannot be used as a key), while dictionary keys need to be immutable (cannot be changed).
If you need to use a list as a key in a dictionary, you should convert it to a tuple before using it as a key.
Can I have multiple values for one key in a dictionary?
No, a dictionary can only store one value for each unique key. However, you can store a list or another collection as the value for a key if you need to have multiple values for a single key.
How do I check if a key exists in a dictionary?
You can use the in
keyword to check if a key exists in a dictionary.
For example:
if "CA" in states:
print("CA exists as a key in states.")
You can also use the .get()
method to check if a key exists in a dictionary. If a key is present in the dictionary, it returns the associated value. Otherwise, it returns None.
value = states.get("CA")
if value is not None:
print("CA exists as a key in states.")
How do I sort a dictionary?
A dictionary is an unordered collection of items and doesn’t have a sort() method like a list. However, you can use the sorted() function along with the items() method to get a sorted list of the dictionary’s items, a list of tuples of key-value pairs.
for key, value in sorted(states.items()):
print(key, value)
or you can sort the keys and access the values using the keys after sorting
for key in sorted(states.keys()):
print(key, states[key])
Keep in mind that if you sort a dictionary, it will only be sorted for the current execution of your script. It will not change the original ordering of the dictionary.