
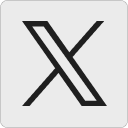




Python developers may be quite familiar with the powerful None keyword. This special constant conveys a value of nothingness or nullity, indicating that no data is present at that point in time. It is an object of its data type, the NoneType
.
In this article, we will take a deep dive into the None
keyword in Python and how it can be used in various contexts. We will also discuss some common pitfalls to avoid when working with None
in your code.
Understanding the None Keyword
Python has a unique keyword known as “None” which is a constant that symbolizes the lack of value or an empty state. It is an object of its data type, the NoneType
.
In Python, None
is not the same as False
, 0
, or an empty string. None
is a distinct object that represents the absence of a value.
Here is an example of how you can use the None
keyword in Python:
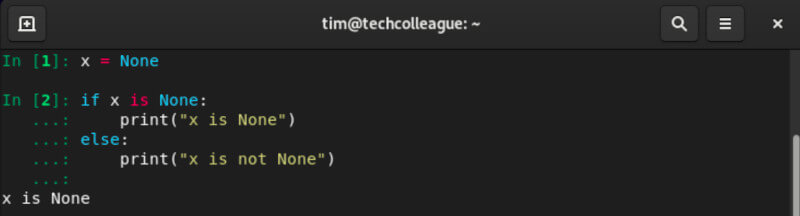
As you can see, the if
statement evaluated to True
because x
is indeed None
.
Using None in Function Arguments
In Python, you can use the None
keyword as a default value for function arguments. This is useful when you want to provide a default behavior for a function argument but you want to allow the user to specify a different value if needed.
Here is an example of a function that takes an optional argument with a default value of None
:
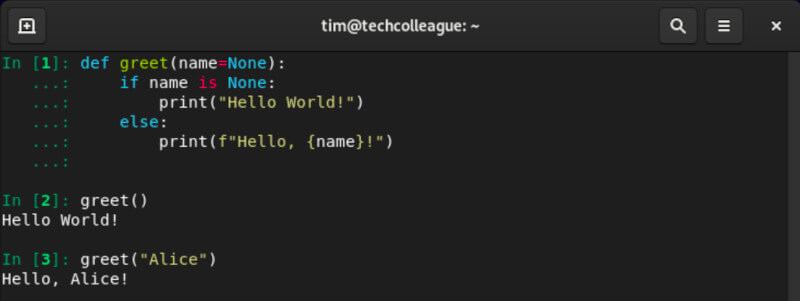
In the example above, the greet()
function takes an optional argument name
with a default value of None
. If the user does not specify a value for name
, the function will use the default value and print “Hello, World!”.
If the user does specify a value for name
, the function will use that value and print “Hello, Alice!” in this case.
Checking for None
In Python, you can use the is
keyword to check if an object is None
. Here is an example:
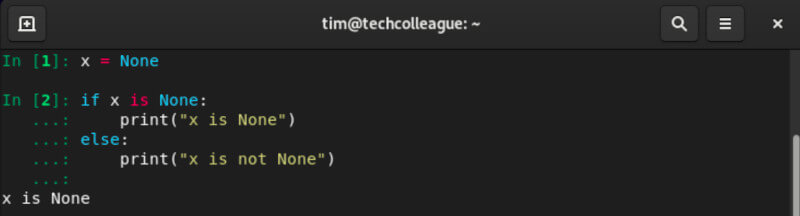
As you can see, the if
statement evaluated to True
because x
is indeed None
.
You can also use the is not
keyword to check if an object is not None
. Here is an example:
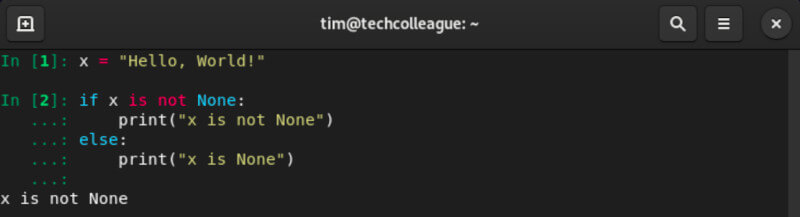
In the example above, the if
statement is evaluated True
because `x` is not None. x evaluates to the variable “Hello, World!”
Handling None in Collections
In Python, you can use the None
keyword to represent a missing value in a collection, such as a list, tuple, or dictionary.
Lists
Here is an example of using None
as a placeholder in a list:
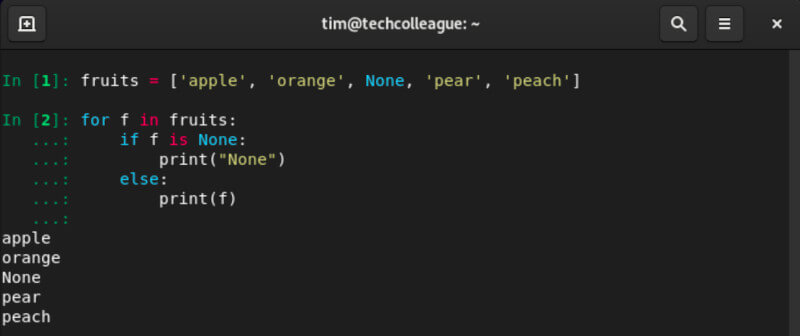
As you can see, the loop printed “None” for the index that contained the None
value.
You can also use the count()
method to count the number of None
values in a list. Here is an example:
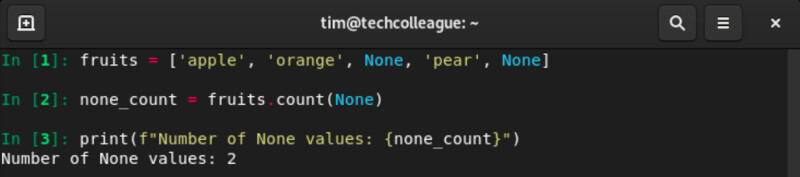
Tuples
You can use the None
keyword in tuples in a similar way as in lists. Here is an example:
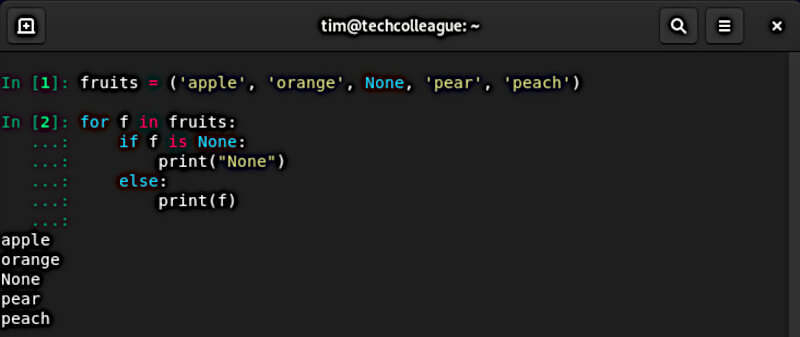
Dictionaries
In dictionaries, you can use None
as the value for a key. Here is an example:
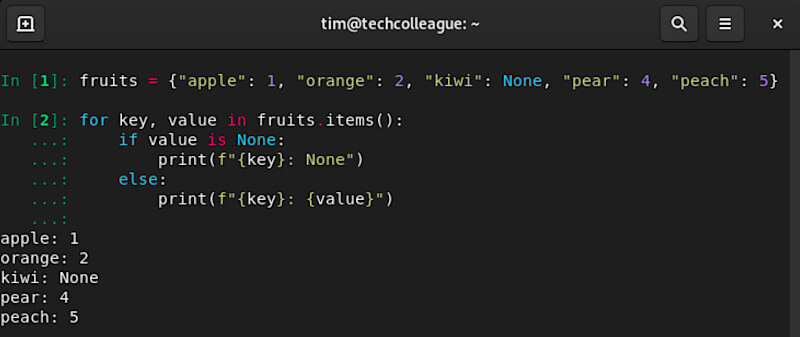
As you can see, the loop printed “None” for the key that had a value of None
.
You can also use the items()
method to filter out the key-value pairs that have a value of None
. Here is an example:
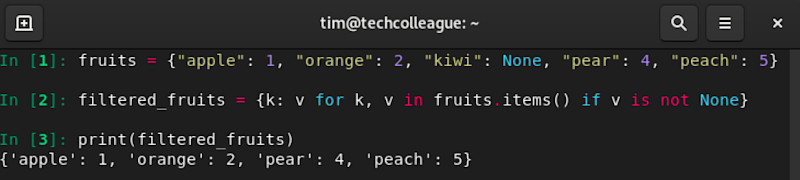
In the example above, dictionary comprehension created a new dictionary with only the key-value pairs that had a value that was not None
.
Common Pitfalls When Working With None
Here are some common pitfalls to avoid when working with None
in your Python code:
Comparing to None using == or !=
In Python, you should use the is
keyword to check if an object is None
, rather than using the ==
or !=
operators. This is because the ==
and !=
operators compare the values of objects, while the is
keyword compares the identity of objects.
Here is an example of using ==
to compare an object to None
:
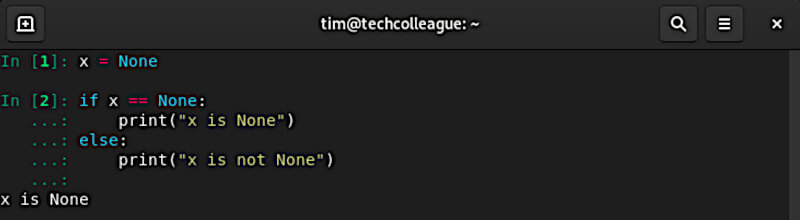
As you can see, the if
statement evaluated to True
because x
is indeed None
. However, using the ==
operator to compare an object to None
is not considered good practice in Python.
The recommended way to check if an object is None
is to use the is
keyword, like this:
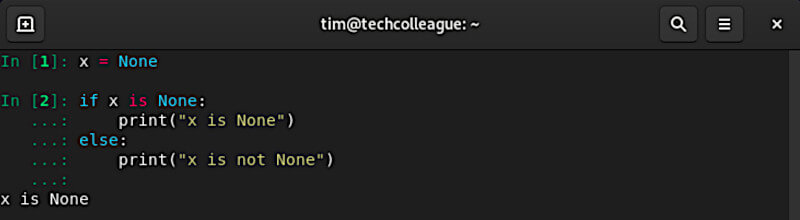
Using the is
keyword ensures that you are comparing the object’s identity, rather than its value.
Assigning None to variables without type hinting
In Python, you can assign the value None
to a variable without declaring its type. However, this can lead to unexpected behavior if you are not careful.
Here is an example of assigning None
to a variable without type hinting:
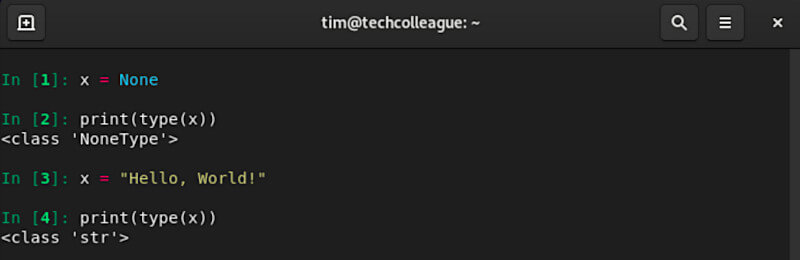
As you can see, the type of the x
variable changed from NoneType
to str
after we assigned a new value to it. Python stands out among other programming languages since it is dynamically typed. This means that the type of a variable is not determined until runtime and only depends on its value assignment.
To avoid this pitfall, you can use type hinting to declare the type of a variable explicitly. Type hinting is a feature in Python that allows you to specify the expected variable type in your code.
Here is an example of using type hinting to declare the type of the x
variable:
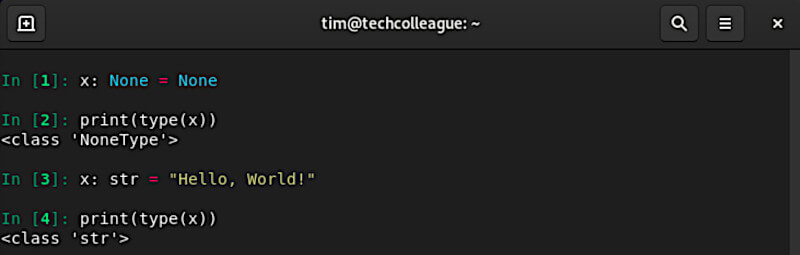
By using type hinting, you can ensure that the x
variable always has the correct type, even if you assign it a different value later on.
Conclusion
In this article, we took a deep dive into the None
keyword in Python and how it can be used in various contexts. We also discussed some common pitfalls to avoid when working with None
in your code.
By understanding the nuances of the None
keyword and how to use it effectively, you can write more concise and reliable Python code.