
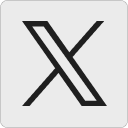




As a Python developer, I’m sure you’ve encountered an error message at some point in your coding journey. One error message that can be particularly frustrating is the “TypeError: ‘list’ object is not callable” message.
In this article, we’ll delve into what this error means and how you can fix it.
What Does the “TypeError: ‘list’ object is not callable” Error Mean?
The Python “TypeError: ‘list’ object is not callable” error appears when invoking a list as if it were a function. In Python, functions are assigned names and perform set tasks – this mistake prevents them from functioning correctly.
Lists, however, are a collection of ordered and changeable items. You can think of a list as an array in other programming languages.
Here’s an example of code that will raise the “TypeError: ‘list’ object is not callable” error:
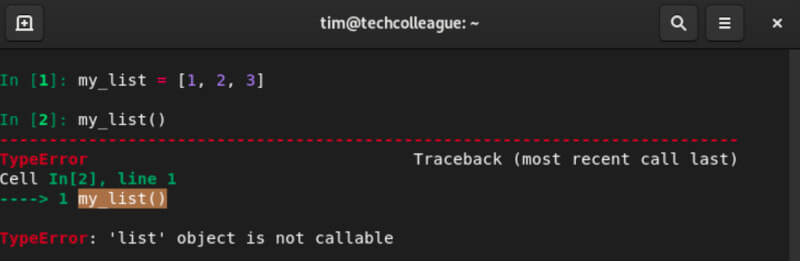
In this code, we define a list called my_list
that contains the elements 1, 2, and 3. Then, we call the list as if it were a function using parentheses. This is not allowed in Python, so the interpreter raises the “TypeError: ‘list’ object is not callable” error.
How to Fix the “TypeError: ‘list’ object is not callable” Error?
One of the primary causes for this issue is that you are attempting to call a list using parentheses. To solve this problem, use square brackets when accessing a specific element from the list.
For example:

In this code, we use square brackets to call the first element in the list.
Another reason this error can occur is that you have assigned a list to a variable with the same name as a built-in function or method in Python.
For example:
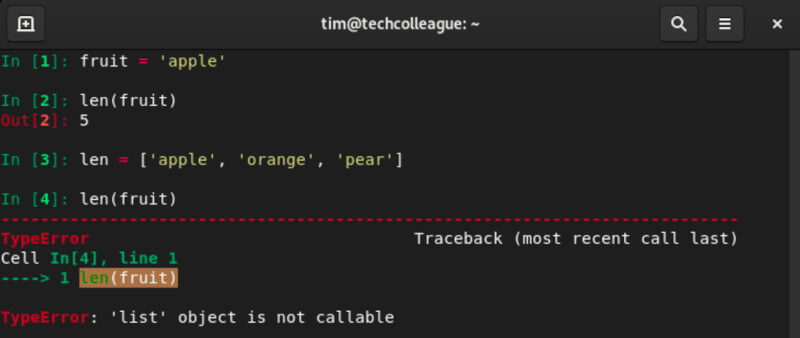
In this code, we assign a list to the variable len
, which is the name of a built-in Python function that returns an object’s length. When we try to call the len
variable as if it were a function, the interpreter raises the “TypeError: ‘list’ object is not callable” error because len
is now a list and not a function. The fix is not to override the built-in Python function.
Here is another example where a built-in function is used:
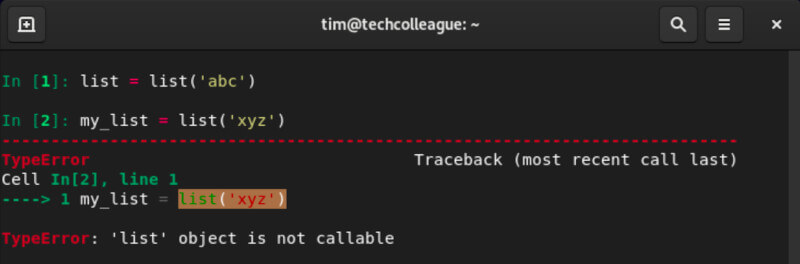
In this code, we assign the variable list
, which is the name of a built-in Python class that returns a list out of an iterable. When we try to assign a variable my_list
to a list, it tries to refer to the list instance we created, but the list is not callable because it is a built-in Python class. The fix is not to override the built-in Python class.
Conclusion
I hope this article has helped you understand the “TypeError: ‘list’ object is not callable” error and how to fix it. Remember, this error occurs when you try to call a list as if it were a function in Python. To fix it, use square brackets when calling a list, and don’t use built-in functions or methods.
If you have any questions or comments, please leave them below.