
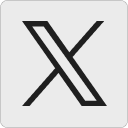




Data type and data structure are two fundamental concepts in computer science and programming. Both are used to organize and manipulate data, but they are different.
In this article, I will explain the difference between data type and structure. I will also go into more detail about some of the most common data types and data structures, along with examples of how they are used in programming.
What is a Data Type?
A data type is a classification of data that defines the type of value that a variable can hold. In other words, it is a way to specify the data type that a variable can store. Some common data types include integers, floating-point numbers, characters, and strings.
For example, if we want to declare a variable that can hold an integer value, we would use the following code:
int x;
In this example, “int” is the data type, and “x” is the variable name. We can then assign a value to the variable “x” like so:
x = 5;
This assigns the value of 5 to the variable “x.” The ” x ” data type is an integer, so it can only hold integer values.
Various Data Types
Integer: An integer is a whole number, either positive or negative. In most programming languages, integers are represented using 4 bytes of memory, which allows for a range of -2,147,483,648 to 2,147,483,647.
Here’s an example of how to declare an integer variable in C++:
int x;
x = 5;
Floating-point: A floating-point number is a number with a decimal point. They are used to represent real numbers using 8 bytes of memory.
Here’s an example of how to declare a floating-point variable in C++:
float y;
y = 3.14;
Characters: A character data type stores a single character, such as a letter or symbol. Characters are usually represented using 1 byte of memory.
Here’s an example of how to declare a character variable in C++:
char z;
z = 'A';
Strings: A string is a sequence of characters. In most programming languages, strings are represented as an array of characters.
Here’s an example of how to declare a string variable in C++:
string name;
name = "John Smith";
What is a Data Structure?
A data structure is a way of organizing and storing data in a specific way. Data structures are used to organize and manipulate data in a specific way. Some common data structures include arrays, linked lists, stacks, and queues.
For example, an array is a data structure that is used to store a collection of data. The following code creates an array of integers:
int numbers[5];
In this example, “numbers” is the name of the array, and “[5]” specifies that the array can hold 5 elements. We can then assign values to the array elements like so:
numbers[0] = 5;
numbers[1] = 8;
numbers[2] = 12;
numbers[3] = 16;
numbers[4] = 20;
This assigns the values 5, 8, 12, 16, and 20 to the elements of the array “numbers.”
Various Data Structures
Array: An array is a data structure that is used to store a collection of data. Elements in an array are stored in contiguous memory locations and can be easily accessed using an index.
Here’s an example of how to declare an array of integers in C++:
int numbers[5];
numbers[0] = 5;
numbers[1] = 8;
numbers[2] = 12;
numbers[3] = 16;
numbers[4] = 20;
Linked List: A linked list is a data structure that is used to store a collection of data in a linear fashion. Each element in a linked list is called a node, containing a reference to the next node. Linked lists are useful when you frequently insert or remove elements from the list.
Here’s an example of how to create a simple linked list in C++:
struct Node {
int data;
Node *next;
};
Node *head;
head = new Node();
head->data = 5;
head->next = NULL;
Stack: A stack is a data structure based on the LIFO (Last In, First Out) principle. This means that the last element added to the stack will be the first one to be removed. Stacks are commonly used in programming for tasks such as undo/redo functionality and keeping track of function calls.
Here’s an example of how to implement a stack in C++ using an array:
#define MAX_SIZE 100
int stack[MAX_SIZE];
int top = -1;
void push(int data) {
if (top == MAX_SIZE - 1) {
cout << "Stack is full" << endl;
return;
}
stack[++top] = data;
}
int pop() {
if (top == -1) {
cout << "Stack is empty" << endl;
return -1;
}
return stack[top--];
}
Queue: A queue is a data structure based on the FIFO (First In, First Out) principle. This means that the first element added to the queue will be the first one to be removed. Queues are commonly used in programming for tasks such as implementing task schedulers and message queues.
Here’s an example of how to implement a queue in C++ using an array:
#define MAX_SIZE 100
int queue[MAX_SIZE];
int front = -1;
int rear = -1;
void enqueue(int data) {
if (rear == MAX_SIZE - 1) {
cout << "Queue is full" << endl;
return;
}
if (front == -1) {
front = 0;
}
queue[++rear] = data;
}
int dequeue() {
if (front == -1) {
cout << "Queue is empty" << endl;
return -1;
}
int data = queue[front++];
if (front > rear) {
front = rear = -1;
}
return data;
}
Conclusion
Data types specify the type of data a variable can hold, while data structures are used to organize and store data in a specific way. They are both important concepts in computer science and programming and are used to manipulate data differently.
These are just a few examples of the many data types and data structures that are used in programming. Understanding the difference between data types and data structures and how to use them effectively is crucial for any programmer.
It is important to note that, depending on the problem you are trying to solve, different data types and data structures may be more appropriate. It’s always good to understand the available options and when to use them for efficient and optimized code.
I hope this article has helped you understand the difference between data type and data structure. Please let me know if you have any questions or need further explanation.
Frequently Asked Questions
When should I use an array over a linked list?
Arrays are better suited for situations where you need to access elements quickly using an index. They are also more efficient for storing a large amount of data. Linked lists, however, are better suited for situations where you need to insert or remove elements frequently.
When should I use a stack over a queue?
Stacks are best used when you implement functionality such as undo/redo or keeping track of function calls, as they are based on the LIFO principle. Queues are best used when you implement functionality such as task schedulers or message queues, as they are based on the FIFO principle.
Are data types and data structures specific to certain programming languages?
No, data types and data structures are fundamental concepts that are used in many programming languages. However, the syntax and implementation of data types and data structures may vary between languages.
How can I improve my understanding of data types and data structures?
Practicing with different data types and data structures and implementing them in different programming challenges and projects can help improve your understanding of them. Reading and studying from reputable sources such as textbooks and online tutorials can also be beneficial.
Are there any other data types and data structures that are not covered in this article?
Yes, there are many other data types and data structures that are not covered in this article such as tree, graph, hash table, etc. Each data structure has its advantages and disadvantages, and it’s important to choose the right one for the task at hand.
It’s always important to remember that the best data type or data structure to use depends on the specific problem you’re trying to solve. It’s good to understand the available options and when to use them to write efficient and optimized code.