
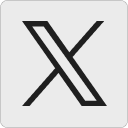




When you see the error message “TypeError: object of type ‘NoneType’ has no len()” in Python, it typically means that you are trying to call the built-in len() function on an object that is of the NoneType, which does not have a length.
This guide will explain this error and how it works and provide examples showcasing a clear illustration of the problem. With our help, you can quickly understand how to resolve the issue in your codebase.
What are some common causes of Python TypeError: object of type ‘NoneType’ has no len()?
Some common causes of this Python error include finding the length of a variable that has not been initialized, trying to get the length of a variable that is None, or trying to get the length of a variable that is not a data structure that has a length property.
This error can also occur when you’re trying to call the len() function on a variable returned by a function or method that did not return any value.
How to fix Python TypeError: object of type ‘NoneType’ has no len()?
- Initialize your variable before trying to find its length.
- Check if the variable is None before trying to find its length, and handle the case where it is None.
- Make sure the variable you’re trying to find the length of is a data structure with a length property.
Here’s an example of a code that will raise this error:
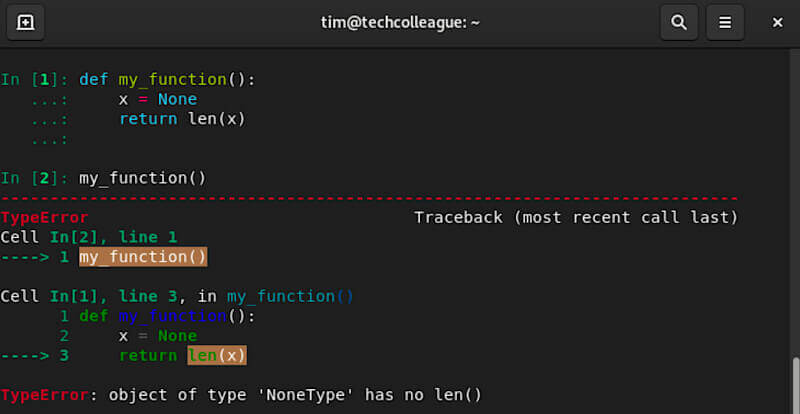
This will raise the error “TypeError: object of type ‘NoneType’ has no len()” because the variable x is None, and thus does not have a length.
Here’s an example of how to fix this error:
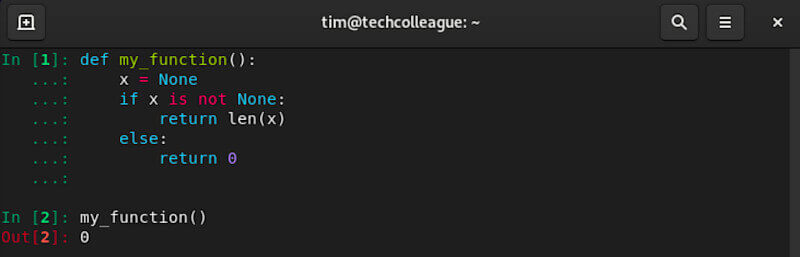
In this example, we first check if the variable x is None before trying to find its length. If it is None, we return 0, otherwise we return the length of x.
Another common cause of the “TypeError: object of type ‘NoneType’ has no len()” error is when you’re trying to call the len() function on a variable that was returned by a function or method that did not return any value.
In Python, if a function or method does not have a return statement or if the return statement is reached without any value being returned, the function will return None.
Here’s an example of this scenario:
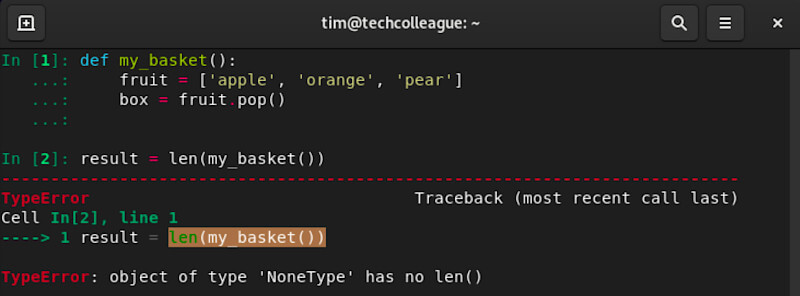
In this example, the my_basket() method calls the pop() method on the list fruit, which removes the last item from the list and returns it. However, my_basket() does not have a return statement, so it returns None. When we try to call len(my_basket()) on the next line, we get the “TypeError: object of type ‘NoneType’ has no len()” error, because None does not have a length.
To fix this, we can add a return statement to the function to return the value of box:
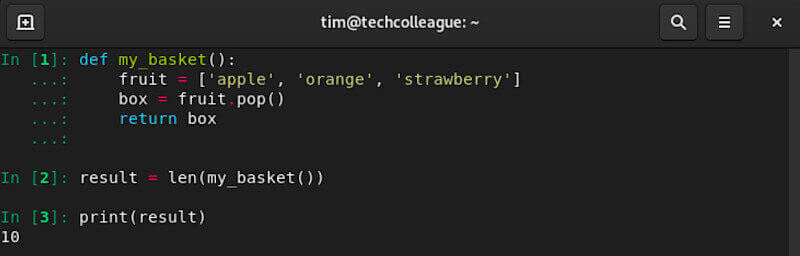
Now, my_basket() will return the value of box, which is the length of the string strawberry.
Conclusion
The “TypeError: object of type ‘NoneType’ has no len()” error occurs when you try to call the len() function on an object that is of the NoneType, which does not have a length.
This error can occur when you’re trying to find the length of a variable that has not been initialized, trying to get the length of a variable that is None, or trying to get the length of a variable that is not a data structure that has a length property.
To fix this error, you can initialize your variable before finding its length, check if the variable is None before trying to find its length, and make sure the variable you’re trying to find the length of is a data structure with a length property.
Frequently Asked Questions
What does the error message “TypeError: object of type ‘NoneType’ has no len()” mean?
The error message “TypeError: object of type ‘NoneType’ has no len()” is raised when you try to call the built-in len() function on an object that is of the NoneType, which does not have a length.
This typically happens when you’re trying to find the length of a variable that has not been initialized, trying to get the length of a variable that is None, or trying to get the length of a variable that is not a data structure that has a length property.
Can this error occur in other programming languages?
This error can occur in other programming languages, although the error message and specific causes may be different. The general concept of trying to find the length of an object that does not have a length property is a common issue in many programming languages.