
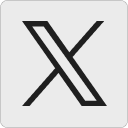




If you’re here, you’re probably wondering what the differences are between HashMap and HashTable. Don’t worry; I’ve got you covered.
HashMap and HashTable are data structures that store and access values using keys. They are both based on hashing algorithms, which means they map keys to their corresponding values using a hash function.
However, while they may seem similar, some key differences can affect their performance and suitability for different use cases.
In this article, we’ll look at these differences so that you can choose the right data structure for your needs.
Key Differences Between HashMap and HashTable
- Thread-safety: HashTable is thread-safe, meaning it can be accessed by multiple threads simultaneously without any issues. However, this comes at the cost of performance since it requires synchronization. HashMap, on the other hand, is not thread-safe by default, but it can be made thread-safe using the ConcurrentHashMap class.
- Performance: HashTable is thread-safe, so it can be slower than HashMap in single-threaded environments. HashTable uses a legacy API, which doesn’t support modern Java features like iterators. HashMap, on the other hand, has better performance in single-threaded environments and supports iterators.
- Null keys and values: Unlike HashTable, which does not accept null keys or values, a HashMap permits one null key and multiple null values.
- Iteration order: HashTable doesn’t maintain any specific order while iterating over the elements, whereas HashMap maintains the order in which elements were added by default.
- Legacy API: HashTable uses a legacy API, which means it doesn’t support modern Java features like iterators, while HashMap uses a modern API.
Understanding these differences will help you choose the right data structure for your needs.
Comparison Table Between HashMap and HashTable
Feature | HashMap | HashTable |
---|---|---|
Implementation | Implemented since Java 1.2 | Implemented since Java 1.0 |
Thread-safety | Not thread-safe by default, but has a thread-safe version (ConcurrentHashMap) | Thread-safe by default, but may suffer from performance issues in multi-threaded environments |
Null values allowed | Allows null values as both keys and values | Does not allow null keys or values |
Iteration order | Does not guarantee any specific order during iteration | Iterates in the order in which elements were added |
Enumeration | Does not support Enumeration | Supports Enumeration for iteration |
Performance | Generally faster than HashTable | May be slower than HashMap in single-threaded environments |
Load factor | Default load factor is 0.75 | Default load factor is 0.75 |
Hashing Algorithm
HashMap and HashTable use a similar hashing algorithm to map keys to their corresponding values. When you insert a key-value pair into the data structure, a hash function generates a hash code for the key.
This hash code is then used to determine the index in the internal array where the value will be stored.
The hash function used in both data structures takes the hash code and applies a bitwise operation to it, which produces a more evenly distributed hash code. This is done to avoid collisions, where two keys generate the same hash code and are stored in the same location in the internal array.
If a collision does occur, both data structures use different techniques to resolve it. HashTable uses chaining, where multiple values with the same hash code are stored in a linked list at the same index.
HashMap uses chaining and open addressing, where the data structure searches for the next empty index to store the value.
The hashing algorithm used in both data structures is designed to be fast and efficient while avoiding collisions as much as possible.
Use Cases
- Use HashMap when you don’t need thread-safety: If you’re working in a single-threaded environment or don’t need thread-safety for your use case, then HashMap is a great choice. It performs better than HashTable in such scenarios and supports modern Java features like iterators.
- Use HashTable when you need thread safety: HashTable is the better choice if your application requires thread safety. It’s designed to be thread-safe and can be accessed by multiple threads simultaneously without issues.
- Use HashMap when you need to store null values: If you need to store null values in your data structure, then HashMap is the better choice. It allows one null key and any number of null values.
- Use HashTable when you need to store legacy code: If you’re working with legacy code that requires using HashTable, you should choose it over HashMap. HashTable uses a legacy API and may be required when modern Java features are not supported.
Understanding your use case is important when choosing between HashMap and HashTable. By considering the needs of your application, you can select the right data structure for your needs.
Best Practices
Here are some best practices to keep in mind when using HashMap or HashTable:
- Use the appropriate data structure for your needs: As discussed earlier, HashMap and HashTable have different use cases, and choosing the right one is important. Consider your application’s requirements, such as thread safety, null values, and iteration order.
- Use the correct hash function: The hash function used by HashMap and HashTable is crucial to their performance. If you’re creating your hash function, ensure it produces evenly distributed hash codes to minimize collisions. Java provides a default hash function for most classes, but you may need to implement your own if using a custom object.
- Beware of concurrent modifications: If you’re using HashMap or HashTable in a multi-threaded environment, be aware of potential concurrent modifications. Both data structures offer thread-safe versions (ConcurrentHashMap for HashMap and synchronized methods for HashTable), but it’s important to use them correctly to avoid issues like deadlocks and performance degradation.
- Use iterators instead of enumeration: If you’re using HashTable, you must use the Enumeration interface to iterate over its elements. However, Enumeration doesn’t support removing elements during iteration. If you use HashMap or need to remove elements during iteration, use the Iterator interface instead.
- Optimize your load factor: The load factor determines how full the data structure can get before it’s resized. A higher load factor means less space is wasted, but it can lead to more collisions and slower performance. A good rule of thumb is to set the load factor to 0.75 for most applications.
By following these best practices, you can ensure that your HashMap or HashTable performs optimally and avoids potential issues.
Conclusion
Choosing between HashMap and HashTable depends on the specific needs of your application. HashMap is a good choice for single-threaded environments and scenarios where null values are needed.
On the other hand, HashTable is designed for multi-threaded environments and legacy code that requires its use.
Both data structures use a hashing algorithm to map keys to their corresponding values, and it’s important to choose the right hash function and load factor to optimize their performance.
Additionally, you should be aware of potential issues like concurrent modifications and choose the appropriate iteration method for your needs.
By understanding the differences between HashMap and HashTable and following best practices, you can ensure that your data structure is performing optimally and meeting the needs of your application.