
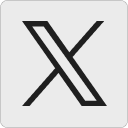




As a software developer, I’m sure you’re familiar with the term “thread safety.” Thread safety is an important concept in software development that ensures multiple threads can access a shared resource without issues or conflicts.
Writing thread-safe code is crucial, as it can help prevent errors and crashes in your application. However, not all code is thread-safe, and using non-thread-safe code can lead to serious problems.
In this article, we’ll explore the differences between thread-safe and non-thread safe code, their advantages and disadvantages, and best practices for writing thread-safe code.
So, let’s dive in!
What is Thread Safe?
Thread safe code is code that can be executed by multiple threads simultaneously without causing any conflicts or issues. This means that even if multiple threads are accessing the same resource, they won’t interfere with each other.
Thread safe code is written in such a way that it ensures consistency and correctness of the shared data.
Developers use synchronization techniques such as locks, mutexes, and semaphores to achieve thread safety. These techniques ensure that only one thread can access the shared resource at any time.
Additionally, thread safe code is designed to minimize the chances of race conditions, deadlocks, and other concurrency issues.
The benefits of using thread safe code are numerous. It helps improve the performance of your application, as multiple threads can work on different parts of the code simultaneously. This can help reduce the time to complete tasks and make your application more responsive.
Furthermore, thread-safe code is more reliable and less prone to errors or crashes, as all threads work together synchronously.
Writing thread safe code requires careful planning and execution, but it’s well worth the effort. By ensuring that your code is thread safe, you can make your application more efficient, reliable, and scalable.
What is Non-Thread Safe?
Non-thread safe code is code that can’t be executed by multiple threads simultaneously without causing conflicts or issues. Multiple threads accessing the same resource can lead to race conditions, deadlocks, and other concurrency issues. This can result in errors or crashes in your application, which can be frustrating for users.
Non-thread safe code doesn’t use synchronization techniques like locks, mutexes, or semaphores to ensure that only one thread can access the shared resource at any given time.
As a result, multiple threads can try to modify or read the same data simultaneously, leading to unpredictable behavior.
Using non-thread safe code can cause problems such as data corruption, inconsistent results, and memory leaks. These issues can be challenging to debug and fix and cause developers significant headaches.
It’s important to avoid using non-thread safe code as much as possible. While writing may be more straightforward, it can lead to serious problems in the long run.
By writing thread safe code, you can ensure that your application is reliable and efficient, and that it can handle multiple threads without any issues.
Thread Safe vs Non-Thread Safe Comparison Table
Thread Safe Code | Non-Thread Safe Code |
---|---|
Uses synchronization techniques like locks, mutexes, or semaphores to ensure that only one thread can access the shared resource at any given time. | Doesn’t use any synchronization techniques, which can lead to race conditions, deadlocks, or other concurrency issues. |
Enables multiple threads to work on different parts of the code simultaneously without causing any conflicts. | May lead to unpredictable behavior, data corruption, and memory leaks due to simultaneous modification of shared resources by multiple threads. |
More reliable and less prone to errors or crashes. | Less reliable and more prone to errors or crashes. |
May be slightly slower due to the overhead of synchronization techniques, but improves the overall performance of the application by enabling multiple threads to work simultaneously. | May be faster in some cases, but can cause significant problems in the long run. |
Ensures consistent and predictable behavior of the code, making it easier to maintain over time. | Can cause unpredictable behavior, making the code difficult to debug and maintain. |
Differences Between Thread Safe and Non-Thread Safe
The main difference between thread safe and non-thread safe code is how they handle access to shared resources. Thread safe code ensures that multiple threads can access the shared resource without any issues, while non-thread safe code doesn’t.
In thread safe code, developers use synchronization techniques like locks, mutexes, or semaphores to ensure that only one thread can access the shared resource anytime. This ensures that multiple threads can work on different parts of the code simultaneously without causing any conflicts.
In contrast, non-thread safe code doesn’t use any synchronization techniques, which can lead to race conditions, deadlocks, or other concurrency issues.
Another key difference between thread safe and non-thread safe code is their reliability. Thread safe code is more reliable and less prone to errors or crashes since it ensures that all threads work together synchronously.
In contrast, non-thread safe code can lead to unpredictable behavior, data corruption, and memory leaks, which can be difficult to debug and fix.
There are also performance differences between thread safe and non-thread safe code. Thread safe code may be slightly slower due to the overhead of synchronization techniques. Still, it can improve the overall performance of your application by enabling multiple threads to work simultaneously.
Non-thread safe code may be faster in some cases, but it can cause significant problems in the long run.
Thread safe and non-thread safe code have advantages and disadvantages, and the choice depends on the specific needs of your application. However, in general, it’s best to err on the side of thread safety whenever possible.
Examples of Thread Safe and Non-Thread Safe Code
Let’s look at some examples of thread-safe and non-thread safe code.
Thread Safe Code Example
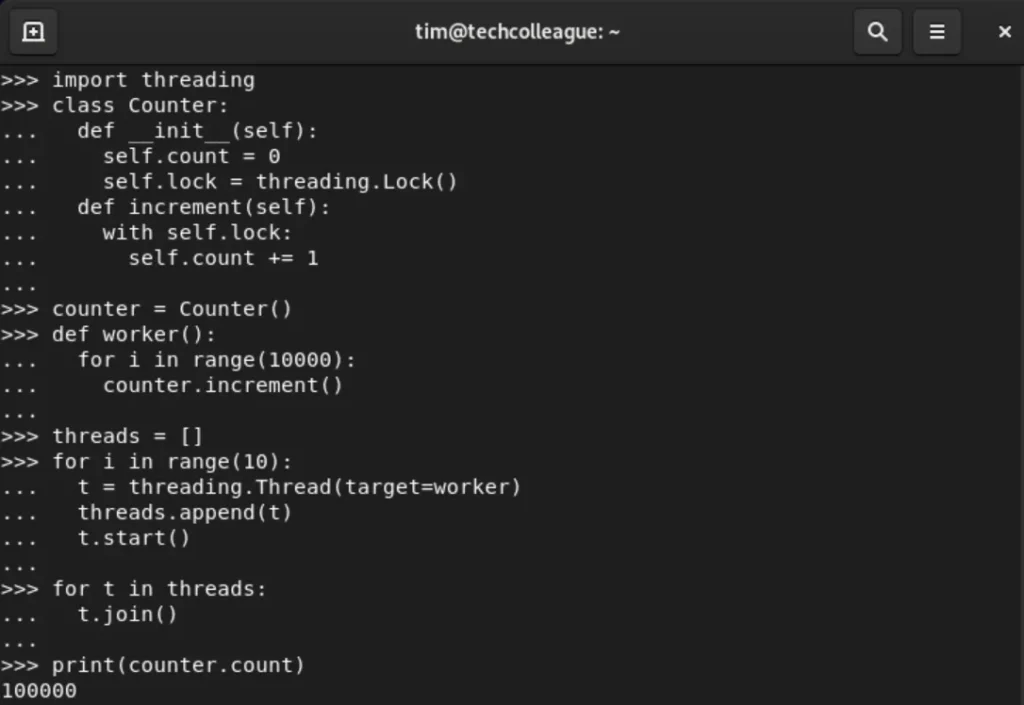
In this example, we use the threading
module to create a counter that multiple threads can access without issues. We define a Counter
class with an internal count and a lock that ensures only one thread can access the count simultaneously.
We then define a worker
function that increments the count 10,000 times, and create 10 threads to execute this function. Finally, we join the threads and print the final count value.
Non-Thread Safe Code Example
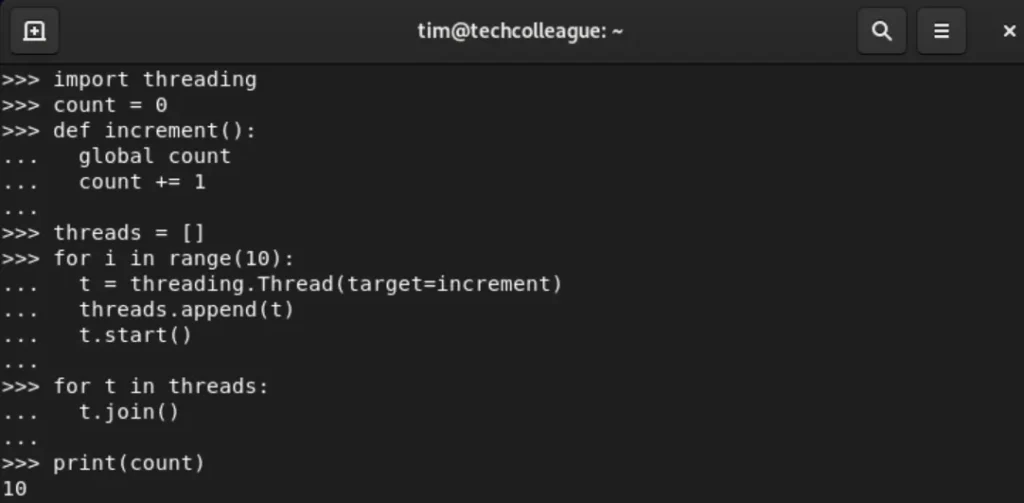
In this example, we have a global count variable that is incremented by a worker
function that runs in 10 threads. Since we don’t use any synchronization techniques like locks or semaphores, the threads may try to modify the count variable simultaneously, leading to unpredictable behavior. As a result, the final count value may not be what we expect.
These examples illustrate the importance of thread safety in ensuring that multiple threads can access shared resources without any issues or conflicts.
Using synchronization techniques like locks, mutexes, and semaphores, you can ensure your code is thread-safe and reliable.
Best Practices for Thread Safety
Writing thread-safe code requires careful planning and execution. Here are some best practices to keep in mind when writing thread-safe code:
- Use synchronization techniques: As we’ve seen, using synchronization techniques like locks, mutexes, and semaphores is crucial to ensuring thread safety. Make sure you use the appropriate synchronization technique for your specific use case.
- Minimize shared state: The more shared state your code has, the more challenging it is to achieve thread safety. Minimize the shared state in your code and only share necessary data.
- Use immutable objects: Immutable objects can’t be modified after creation. Using immutable objects can help simplify your code and make it easier to reason about, which can help achieve thread safety more easily.
- Avoid deadlocks: When multiple threads are blocked and waiting for each other to release a resource, that’s what’s known as a deadlock. To avoid deadlocks, ensure that your threads always acquire resources consistently.
- Test your code thoroughly: It is crucial to ensure it’s thread-safe. Write comprehensive unit tests that cover all possible scenarios and edge cases.
- Follow best practices for concurrency: Finally, ensure you follow best practices for concurrency in general. This includes avoiding busy waiting, minimizing contention, and minimizing context switching.
By following these best practices, you can improve the quality of your code and make it easier to maintain over time. Remember, thread safety is crucial to ensure reliable and efficient code, so take the time to write thread-safe code whenever possible.
Conclusion
Thread safety is a crucial concept in software development that ensures multiple threads can access a shared resource without issues or conflicts.
Writing thread-safe code requires careful planning and execution, but it’s worth the effort. By ensuring that your code is thread-safe, you can make your application more efficient, reliable, and scalable.
Remember, thread-safe code uses synchronization techniques like locks, mutexes, and semaphores to ensure that only one thread can access the shared resource anytime.
Additionally, it’s essential to minimize shared state, use immutable objects, and avoid deadlocks when writing thread-safe code. Finally, thorough testing and following best practices for concurrency are crucial to ensuring the quality of your code.
Understanding thread safety and writing thread-safe code is essential for any software developer. By keeping these concepts and best practices in mind, you can ensure that your code is reliable, efficient, and ready for any challenges that come your way.