
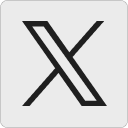




Have you ever encountered the problem of importing modules from a parent directory in Python? It can be pretty frustrating when you have to deal with complex project structures and you need to import modules from a higher-level directory.
I want to share some tips and tricks on importing modules from a parent directory in Python.
Importing modules from a parent directory is essential when you want to reuse code or when you need to work with modules that are not part of your current project. Understanding how to import modules from a parent directory is crucial for Python developers, and it can save a lot of time and effort.
In this article, we’ll explore the different methods to import modules from a parent directory in Python. We’ll also discuss best practices for organizing modules and packages to avoid the need for importing from a parent directory.
By the end of this article, you’ll better understand how to import modules from a parent directory in Python and how to follow best practices when doing so.
Background
Before we dive into how to import modules from a parent directory in Python, let’s briefly go over the basics of Python’s module system. In Python, a module is a file containing Python definitions and statements.
When you import a module, you run the code in that file.
When you import a module, Python searches for it in a specific order. It first looks in the directory of the script that’s currently running. If it doesn’t find the module, it looks in the directories specified in the PYTHONPATH environment variable. Finally, it looks in the standard system directories.
Python offers two types of imports: relative and absolute imports. Relative imports are used when importing a module that’s in the same package as the importing module. Absolute imports are used when importing a module that’s not in the same package as the importing module.
Now, let’s move on to the main topic of this article: importing modules from a parent directory in Python. This is necessary when you have modules in a higher-level directory to use in your current project.
There are a few different methods to accomplish this, so let’s explore them in more detail.
Importing Modules From A Parent Directory
When importing modules from a parent directory in Python, there are a few methods to choose from. The first method is to use the sys.path.append() function adds the parent directory to the module search path. This allows Python to find and import modules from the parent directory.
Here’s an example:
import sys
sys.path.append('../parent_directory')
import module_from_parent_directory
The second method involves using the package structure. This method is more flexible and allows for more organized code. To use this method, you must create a package in the parent directory and add an init.py file.
Here’s an example:
project_directory/
parent_directory/
__init__.py
module_from_parent_directory.py
main.py
In the init.py file, you can import the module(s) you need from the parent directory:
from parent_directory.module_from_parent_directory import function_from_module
Then, in the main.py file, you can import the function(s) you need from the parent directory package:
from parent_directory import function_from_module
Suppose I have a project directory that looks like this:
my_project/
parent_directory/
__init__.py
helper_functions.py
main.py
In the helper_functions.py
file, I have some useful functions that I want to use in my main.py
file. Here’s how I would import the helper_functions
module into my main.py
file:
# Importing modules from a parent directory
import sys
sys.path.append('../parent_directory')
from parent_directory.helper_functions import techcolleague_method
# Now I can use the techcolleague_method in my main code
techcolleague_method()
In this example, I’m using the sys.path.append()
method to add the parent directory to the Python module search path. This allows Python to find and import the helper_functions
module from the parent directory.
Alternatively, I could use the package structure method to import the helper_functions
module from the parent directory.
Here’s what that would look like:
# Importing modules from a parent directory using package structure
from parent_directory import helper_functions
# Now I can use the useful_function in my main code
helper_functions.techcolleague_method()
In this example, I’ve created a package called parent_directory
in the parent directory and added an __init__.py
file to it. This allows me to import the helper_functions
module using the package structure method, which is more organized and maintainable.
In the first example, I’m using the sys.path.append()
method to add the parent directory to the Python module search path. This method appends the parent directory to the list of directories Python searches for modules when encountering an import statement. This allows Python to find and import the helper_functions
module from the parent directory.
In the second example, I’m using the package structure method to import the helper_functions
module from the parent directory. This method involves creating a package in the parent directory and adding an __init__.py
file to it.
The __init__.py
file tells Python that the directory should be treated as a package and allows us to import modules using the package structure method. This method is more organized and maintainable, especially for larger projects.
Importing modules from a parent directory in Python can be useful when done correctly. It allows us to reuse code across multiple files and keep our project organized.
However, it’s important to follow best practices and avoid potential issues, such as modifying the PYTHONPATH environment variable. Using relative imports, organizing our code into packages and modules, and using a virtual environment can make our code more maintainable and efficient.
Related: Python Extract Number From String
Best Practices For Importing From A Parent Directory
While it’s sometimes necessary to import modules from a parent directory in Python, it’s generally a good idea to avoid it if possible.
Here are some best practices to follow when importing from a parent directory:
- Use relative imports instead of absolute imports whenever possible. Relative imports make it easier to move code around without breaking import statements.
- Organize your code into packages and modules that reflect the logical structure of your project. This can help reduce the need to import modules from a parent directory.
- Use a virtual environment to isolate your project dependencies. This can help prevent conflicts between different versions of the same module.
- Avoid modifying the PYTHONPATH environment variable. This can lead to hard-to-debug issues and make it difficult for other developers to run your code.
- Choose a clear and maintainable method if you must import modules from a parent directory. Document your imports clearly and consistently to make it easy for other developers to understand.
Following these best practices can help you avoid the need to import modules from a parent directory in Python and make your code easier to maintain and understand.
Conclusion
Importing modules from a parent directory in Python can be useful, but using it wisely and following best practices to avoid potential issues is important. By using relative imports, organizing your code into packages and modules, using a virtual environment, and avoiding modifications to the PYTHONPATH environment variable, you can make your code more maintainable and easier to understand.
Remember, there are multiple ways to import modules from a parent directory, so choose the method that best fits the structure of your project and the needs of your code. Following these best practices and staying organized can make your code more efficient and effective.