
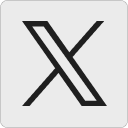




Have you ever needed to extract numbers from a string in Python? It can be a common problem when working with text data, especially in data cleaning and analysis tasks.
In this article, we’ll explore three different Python methods for extracting numbers from a string.
We’ll discuss the advantages and disadvantages of each approach and provide some code snippets to help you implement them in your projects.
Using Regular Expression
One of the most powerful and flexible ways to extract numbers from a string in Python is by using regular expressions. Regular expressions, or regex for short, are a pattern-matching language that allows you to search for specific patterns in text.
To use regex for extracting numbers, we’ll need to create a pattern that matches any string of digits in the text.
Creating a regex pattern for extracting numbers in Python is straightforward. We can use the \d
character class, which matches any digit, and the +
quantifier, which matches one or more occurrences of the preceding character.
So, our pattern to match any string of digits would be \d+
. We can then use this pattern with the re.findall()
function in Python to extract all occurrences of the pattern in a string.
Here’s an example of how to use regular expressions to extract numbers from a string in Python:
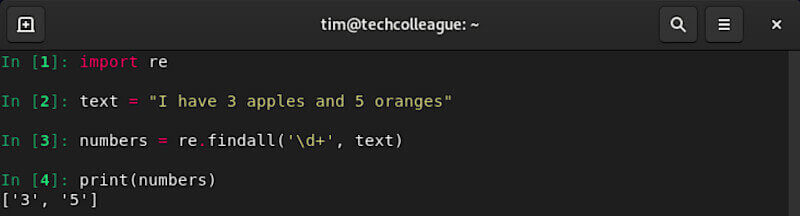
In this example, we first imported the re
module, which provides support for regular expressions in Python. We then defined a string called text
that contains some text and numbers.
Finally, we used the re.findall()
function to extract all occurrences of the \d+
pattern in the text
string, which gave us a list of the numbers as strings.
Regular expressions can be a powerful way to extract numbers from strings in Python, especially if you need to extract numbers with specific patterns or formatting.
However, it requires some knowledge of regular expressions, which can be challenging.
Using isdigit() Method
Another way to extract numbers from a string in Python is by using the built-in isdigit()
method. This method is a simple approach to extracting all digits from a string.
The isdigit()
method checks whether all the characters in a string are digits or not. If all the characters are digits, then it returns True
. Otherwise, it returns False
.
Here’s an example of how to use the isdigit()
method to extract numbers from a string in Python:
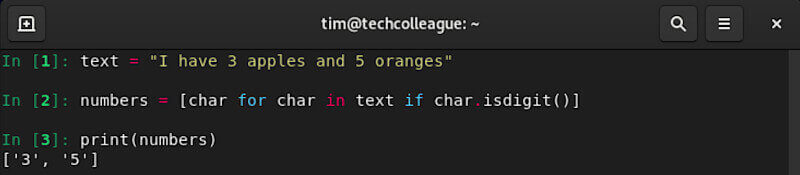
In this example, we defined a string called text
that contains some text and numbers. We then used a list comprehension to iterate over each character in the text
string and checked if it was a digit or not using the isdigit()
method. If the character is a digit, we add it to the numbers
list.
Using the isdigit()
method can be a quick and easy way to extract numbers from a string in Python. However, it only works if the string contains only digits and no other characters.
If your string contains non-digit characters, you must use a different method.
Related: Python Import From Parent Directory
Using split() Method
A third way to extract numbers from a string in Python is by using the built-in split()
method. This method splits a string into a list of substrings based on a specified delimiter.
We can use this method to split a string into a list of words and then iterate over the words to check whether each word is a number.
Here’s an example of how to use the split()
method to extract numbers from a string in Python:
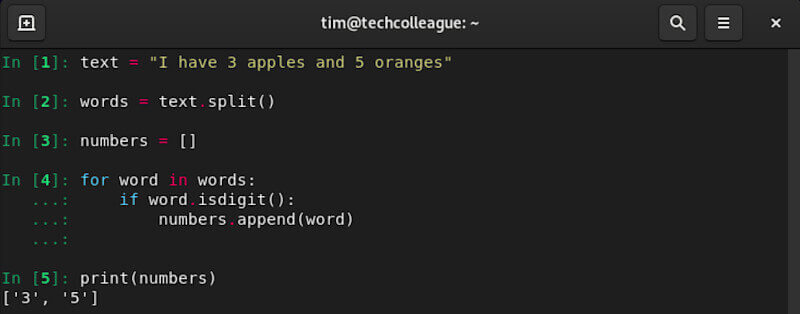
In this example, we first defined a string called text
that contains some text and numbers. We then used the split()
method to split the text
string into a list of words.
Finally, we iterated over each word in the words
list and checked if it was a digit or not using the isdigit()
method. If the word is a digit, we add it to the numbers
list.
Using the split()
method can be a simple and effective way to extract numbers from a string in Python. However, it relies on properly formatting the string with spaces or other delimiters between words. If your string is not well-formatted, this method may not work as intended.
Conclusion
This article explored three different Python methods to extract numbers from a string. We covered using regular expressions, the isdigit()
method, and the split()
method.
Each method has advantages and disadvantages, and the best approach depends on the specific requirements of your task.
Regular expressions may be the best option to extract numbers from a string with specific patterns or formatting. The isdigit() method is simple but only works if the string contains only digits.
Finally, the split()
method can effectively extract numbers if the string is well-formatted with spaces or other delimiters.
Using these methods, you can extract numbers from strings in Python and use them for various purposes such as data analysis, data science, or natural language processing.
With some practice and experimentation, you’ll easily choose the best method for your task and extract numbers from strings.