
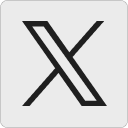




As a programmer, I often find myself working with lists in Python. One of the most common tasks I encounter is concatenating multiple lists into a single one.
In this article, I will share with you different methods of how to concatenate lists in Python.
What is Concatenation in Python?
In Python, concatenation combines two or more strings or lists into a single one. When we concatenate two lists, we are essentially merging them into one. Concatenation in Python is achieved using various methods, as discussed in this article.
To better understand how concatenation works, let’s look at an example:
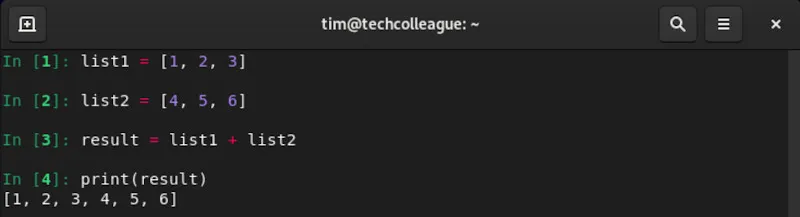
In this example, we have two lists, list1 and list2. We then concatenate them using the “+” operator, which results in a new list containing all the elements from both lists.
Concatenate Lists Using “+” Operator
The “+” operator is one of the most straightforward methods of concatenating lists in Python. To use this method, we use the “+” operator to combine two or more lists into a new one.
Here’s an example:
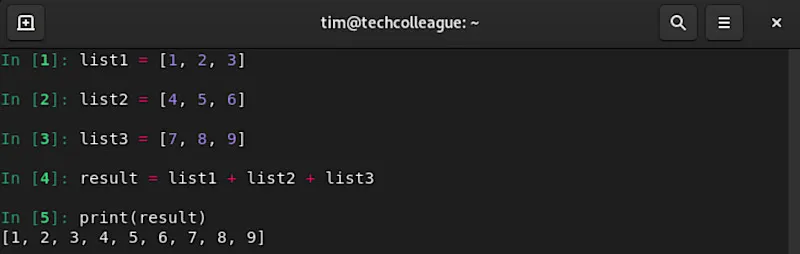
In this example, we have three lists: list1, list2, and list3. We then concatenate them using the “+” operator, which results in a new list containing all the elements from all three lists.
Related: Python Extract Number From String
Concatenate Lists Using “extend()” Method
The “extend()” method is another way to concatenate lists in Python. This method is handy when we want to add elements from one list to another.
Here’s an example:
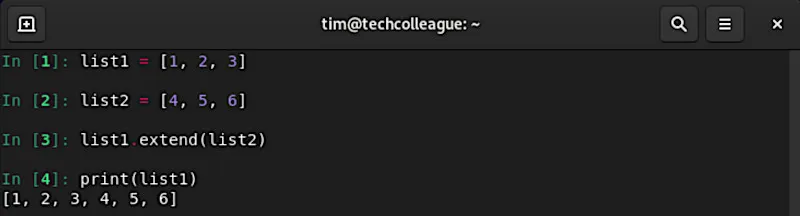
In this example, we have two lists, list1 and list2. We then use the “extend()” method to add all the elements from list2 to list1.
Concatenate Lists Using “append()” and “join()” Methods
The “append()” and “join()” methods are also useful when it comes to concatenating lists in Python. The “append()” method adds a single element to the end of a list, while the “join()” method combines a list of strings into a single string.
Here’s an example:
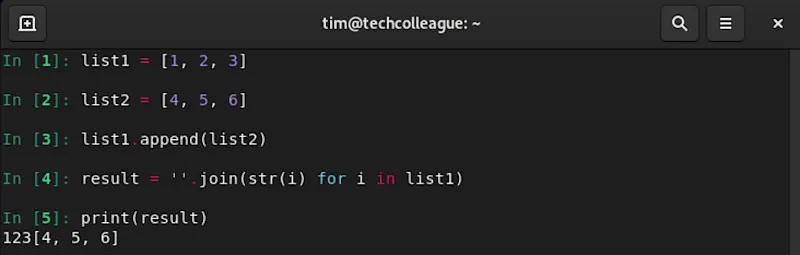
In this example, we have two lists: list1 and list2. We then use the “append()” method to add list2 as a single element to list1. We then use the “join()” method to combine all the elements in list1 into a single string.
As you can see, the “append()” method adds list2 as a single element to list1, and the “join()” method combines all the elements in list1 into a single string.
Concatenate Lists Using “chain()” Function from itertools Module
The “chain()” function from the itertools module is another method for concatenating lists in Python. This function takes multiple iterables as arguments and returns a single iterable that contains all the elements from all the iterables.
Here’s an example:
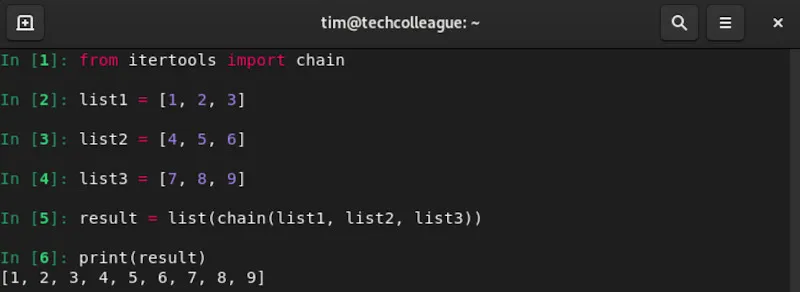
In this example, we import the “chain()” function from the itertools module. We then have three lists: list1, list2, and list3.
We pass all three lists as arguments to the “chain()” function, which returns a single iterable that contains all the elements from all three lists. We then convert this iterable back into a list using the “list()” function.
Conclusion
We have explored various methods of how to concatenate lists in Python. We have seen that there are multiple ways to achieve this task, depending on the requirements of the project.
By using the “+” operator, “extend()” method, “append()” and “join()” methods, and the “chain()” function from the itertools module, we can easily concatenate multiple lists into a single one.
With this knowledge, we can now handle the task of concatenating lists in Python with ease.