
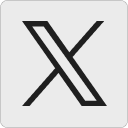




When you need to combine two strings in Bash, there are a few different ways to do it. We will go over all of the options so that you know what your best option is in any given situation.
In this blog post, we’re going to explore the Bash concatenate string operator. This is a very useful operator that allows you to join two or more strings together into one.
Option 1: Adding Two Variables Together
This is the simplest option. If you just need to add two strings together, then add two strings together without any spaces between them.
VAR1="Hello"
VAR2="World"
echo "$VAR1$VAR2"
HelloWorld
If you want to save the concatenated strings to another variable, you can assign them to a third variable.
VAR1="Hello"
VAR2="World"
VAR3="$VAR1$VAR2"
To print out the output of VAR3 after two variables have been concatenated, run the following:
echo $VAR3
HelloWorld
Option 2: Use printf and the Format Specifier
Another option is to concatenate two strings using the printf command and the format specifier (%s).
To see it in action, run this command:
VAR1="Hello"
VAR2="World"
echo $(printf "%s" "$VAR1$VAR2")
HelloWorld
You can further enhance this command by adding your own formatting from within the printf command.
VAR1="Hello"
VAR2="World"
echo $(printf "%s there, %s." "$VAR1" "$VAR2")
Hello there, World.
Option 3: Using the “+=” Operator
In Bash, you can use the “plus equal signs” operator to combine two strings. The way this works is by taking a variable and appending it to another variable.
VAR1="Hello"
VAR1+="World"
echo $VAR1
HelloWorld
If you have a few strings that you want to concatenate, you can do so using the for loop command.
BASKET=""
for FRUIT in apple orange strawberry banana peach; do
BASKET+="$FRUIT "
done
echo $BASKET
apple orange strawberry banana peach
How to Concatenate Numbers in Bash
Similar to strings, you can concatenate numbers in Bash. However, the output will be very different. Rather than concatenate the numbers, Bash will get the sum total of the numbers.
VAR1=5
VAR2=7
echo $((VAR1+VAR2))
12
As you can see above, you get 12 rather than 57. If you intend to really concatenate the numbers together, you can run the same command as Option 1.
VAR1=5
VAR2=7
echo "$VAR1$VAR2"
57
Conclusion
We hope this tutorial has helped you learn how to concatenate strings in Bash. If you have any feedback, let us know.
Also, be sure to check out our other tutorials on Bash Split String Examples and Automation with Bash Scripts if you’re interested in learning more about these topics.