
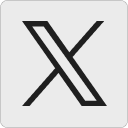




In bash scripting, due to the lack of any built-in string function, it is exceptionally challenging for a programmer to split the string data in bash. It requires the usage of various bash built-in commands and functions so that some specific characters are removed from the string and successively get executed.
For the purpose of splitting a single string data into multiple parts, many programmers use a comma as a delimiter.
But many programmers are not aware of different methods to split the complex and large strings. In this article, I will describe some bash split string examples for you.
Using the IFS Variable
The Internal Field Separator (IFS) variable is available by default in bash scripts. This IFS variable is used for splitting a string into words. Word boundaries are identified by this IFS value.
It can be changed selectively based upon the requirement of the script. The variable is set to equal to the space character by default, so any other characters, like TAB, CR/LF, etc will be treated as word separators and added as elements.
Bash provides the IFS variable for working with strings. It is used to split the command arguments into separate words. A word can be a part of a string separated by any white space.
Example 1: Split String Based on a Space
Suppose you have a sentence that you want to split based on a space. You can assign the space to the IFS variable and loop through the sentence.
split_example1.bash
#!/bin/bash
SENTENCE="Life is what happens when you're busy making other plans by John Lennon"
# Assign another variable OLD_IFS to IFS so you can set it back later.
OLD_IFS=$IFS
# Set space as the delimiter.
IFS=' '
# Loop through the sentence.
for WORD in $SENTENCE
do
echo "${WORD}"
done
# Assign the variable IFS back to the original value.
IFS=$OLD_IFS
Run the script split_example1.bash.
bash split_example1.bash
The output of split_example2.bash is shown below:
Life
is
what
happens
when
you're
busy
making
other
plans
by
John
Lennon
Example 2: Split String Based on a Semicolon
Suppose you have a string of email addresses separated by a semicolon. How would you separate it? You can do so by defining the semicolon for the IFS variable.
split_example2.bash
#!/bin/bash
EMAILS="[email protected];[email protected];[email protected]"
# Assign another variable OLD_IFS to IFS so you can set it back later.
OLD_IFS=$IFS
# Set semicolon as the delimiter.
IFS=';'
# Loop through the email addresses.
for EMAIL in $EMAILS
do
echo "${EMAIL}"
done
# Assign the IFS variable back to the original value.
IFS=$OLD_IFS
Run the script split_example2.bash.
bash split_example2.bash
The output of split_example2.bash is shown below:
[email protected]
[email protected]
[email protected]
Example 3: Split String Based on Multiple Characters
It’s possible your sentence may contain repeating characters such as colons between words. You can use these repeating characters as a way to split them up but it will be a bit more involved. Setting the IFS variable to the colon is not enough since the number of colon characters may vary.
split_example3.bash
#!/bin/bash
SENTENCE="The::journey:::of::a::thousand::miles::::begins::with:one::step::by:Lao:::Tzu"
# Assign another variable OLD_IFS to IFS so you can set it back later.
OLD_IFS=$IFS
# Set colon as the delimiter.
IFS=':'
ARR=(${SENTENCE})
# Set IFS variable to an empty string.
IFS=""
ARR=(${ARR[@]})
# Loop through each word in the sentence.
for ((i=0; i < ${#ARR[@]}; i++)); do
echo "${i}: ${ARR[${i}]}"
done
# Assign the variable IFS back to the original value.
IFS=$OLD_IFS
Run the script split_example3.bash.
bash split_example3.bash
The output of split_example3.bash is shown below:
0: The
1: journey
2: of
3: a
4: thousand
5: miles
6: begins
7: with
8: one
9: step
10: by
11: Lao
12: Tzu