
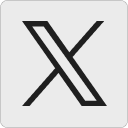




As a programmer, I’ve always been fascinated by the intricacies of different programming languages, and Python is no exception. It’s one of the most popular languages, known for its readability, simplicity, and wide range of uses—from web development to data analysis and machine learning.
One of the things that I find truly fascinating about Python is its functions. They’re the building blocks of any Python program, allowing us to encapsulate a task in a single, reusable code unit. This makes our programs more organized, efficient, and easily understood.
But here’s the thing: not all functions behave the same way. Two of Python’s function-related keywords—return and yield—can affect how a function behaves differently.
In this article, we’ll dive deep into these two keywords, understanding their meaning, how they work, and when to use each. Whether you’re a Python newbie or a seasoned pro, I hope this discussion will shed some light on this important subject and help you write better, more efficient code.
Let’s get started!
Understanding Python’s Return Statement
When I first started coding in Python, I found the return keyword slightly mysterious. It seemed to have some magical power to make a function spit out any value I wanted. But as I delved deeper into Python programming, I realized that return it is not as mysterious as it seems—it’s a very useful tool that can help us control the flow of our programs.
In its simplest form, the return statement is like the final bow of a function—the moment it exits the stage and hands over a result to the part of the program that called it. When a function encounters a return statement, it immediately stops what it’s doing and sends the specified value back to the caller.
Here’s a simple example:
In this code, add_numbers is a function that takes two arguments, a and b, adds them together, and then uses the return statement to give the result back to the caller. When we call this function with the numbers 5 and 7, it returns the value 12, which we print out.
But the return statement has more tricks up its sleeve. For one thing, it doesn’t have to return a value at all. If a function doesn’t need to give anything back to the caller, it can simply use a bare return statement like this:
In this function, the return statement is just used to signal that the function is done and there’s no further code to execute.
The return statement is a powerful tool for controlling a function’s behavior. It allows a function to produce a result that can be used elsewhere in your program and gives you precise control over where and when your function ends.
As you continue your Python journey, you’ll find it invaluable to your coding toolkit.
Understanding Python’s Yield Statement
When I first encountered the yield keyword in Python keyword in Python. It seemed similar to return, but not quite the same. As I began to unravel its mysteries, I discovered that yield is a powerful tool for creating generators in Python.
Generators are iterable, like lists or tuples. But unlike lists and tuples, generators don’t store all their values in memory. Instead, they generate each value on the fly as you loop over them. This makes them incredibly memory-efficient, especially when dealing with large data sets.
Here’s where yield comes in: the keyword makes generators possible. When a function encounters a yield statement, it returns the specified value to the caller – just like a return. But here’s the twist: instead of terminating the function, yield pauses it.
The function’s state (including local variables) is saved, and execution is returned to the caller. The next time the function is called, it resumes right where it left off.
Let’s look at an example:
Count_up_to is a generator function in this code that counts from 1 up to n. Each time it encounters the yield statement, it yields the current i value to the caller, then pauses. When we loop over the generator, it resumes counting where it left off until it has counted up to n.
The yield statement is like a pause button for functions, making it easy to create efficient, stateful, custom-made iterators. It’s a concept that can take a little getting used to, but once you’ve got the hang of it, you’ll find it’s a powerful addition to your Python programming toolbox.
Differences Between Yield and Return
Firstly, yield and return are used to give value back to the caller. However, what happens next is what sets them apart.
When a function encounters a return statement, it stops execution and sends the specified value back to the caller. After a function returns, it’s done—it has no memory of its previous state.
On the other hand, when a function encounters a yield statement, it also returns the specified value—but it doesn’t forget where it left off. The function pauses its execution, and the next time you call it, it picks up right where it left off, with all its local state intact.
Here’s a simple way to think about it: return is like a sprinter who runs a race as fast as possible, then completely stops. On the other hand, Yield is like a long-distance runner who paces herself, taking breaks (or yielding) at regular intervals but always ready to pick up where she left off.
This difference is particularly important when dealing with large data sets. A function that returns a list needs to generate all the elements upfront, which can take time and memory. But a generator function that uses yield produces elements one at a time, on-demand, which is much more memory-efficient.
So, return hands a value and terminates the function while yield produces a stream of values over time, pausing the function between each one. Both are essential tools in your Python toolkit and understanding when to use each can make your code more efficient and easier to read.
Similarities Between Yield and Return
First off, both yield and return are used within functions. They’re tools that a function uses to communicate with the outside world. When your function has computed a value that needs to be sent back to the code called the function, yield and return are your go-to guys.
Secondly, yield and return produce a value that gets passed back to the caller. When your function encounters a yield or return statement, it immediately sends the specified value to wherever the function was called. In other words, yield and return can spit out values from a function.
Here’s an example:
In both cases, the function produces a string that gets printed out.
So, despite their differences, yield and return have much in common. They’re both ways for a function to produce a result that can be used elsewhere in your code. Understanding these two powerful tools is key to mastering function writing in Python.
Key Takeaways
Both yield and return are used within functions: These are tools a function uses to communicate with the outside world. They’re like the function’s voice, letting it express the results of its computations to the rest of your code.
- They both produce values: When your function has crunched the numbers (or strings, or lists, or whatever it’s working with) and it’s time to send a result back to the caller, yield and return are how it does it.
- The difference lies in what happens next: After a return statement, the function is done. It forgets what it was doing and starts from scratch the next time you call it. But after a yield statement, the function takes a break, remembering where it left off and ready to pick up again when needed.
- Generators are key: The yield keyword is used to create generator functions. These special kind of iterable generate their elements on the fly.
- Memory Efficiency: If you’re dealing with large data sets, generators (and therefore yield) can be your best friend due to their memory efficiency.
Yield and return: They might seem similar at first, but they have distinct roles in your Python code. Understanding these two can help you write more efficient and effective Python code, whether you’re sprinting with return or pacing yourself with yield.
Conclusion
Understanding Python’s nuances in yield and return has been enlightening. While they both serve to produce results from a function, their behavior beyond that point is what sets them apart.
Return is your one-and-done tool. It gives a result back to the caller and then washes its hands of the situation. It’s like a sprinter who runs full tilt toward the finish line and then stops.
On the flip side, yield is more of a marathon runner. It produces a result, but instead of stopping, it takes a breather, preserving its state for the next round. This makes yield especially useful when dealing with large data sets where memory efficiency is key.
In the end, yield and return have unique strengths and uses. They are both fundamental aspects of Python, and understanding them enhances your coding skills and opens up new possibilities in how you approach problem-solving in Python.
So, whether you’re sprinting with return or pacing with yield, remember coding is not just about reaching the finish line. It’s about enjoying the race.