
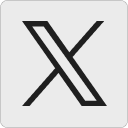




Have you ever been working on a Python program, only to come across the error message “TypeError: not enough arguments for format string”? As a Python programmer, I’ve encountered this error numerous times, and it can be frustrating to figure out what’s causing it and how to fix it.
In this article, I’ll explain what this error message means, the common causes of the error, and provide some solutions and best practices for avoiding it in the future.
So, if you’re ready to dive into the Python string formatting world, let’s get started!
Understanding the TypeError: not enough arguments for format string error
So, you might be wondering, what does this error message mean? Simply put, it means that you’re trying to format a string using the .format() method, but you haven’t provided enough arguments to fill all the placeholders in the string.
For example, let’s say you have a string with two placeholders like this:
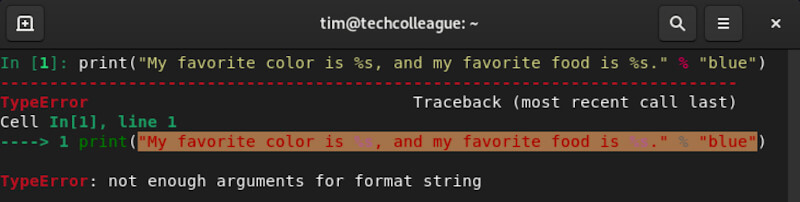
You’ll get the “TypeError: not enough arguments for format string” error because you haven’t provided enough arguments to fill both placeholders.
This error can be confusing because it doesn’t tell which placeholders are missing arguments. However, if you look at the code causing the error, you should be able to figure out which placeholders need to be filled.
In the next section, we’ll look at some common causes of this error, so you can better understand how to diagnose and fix it.
How to Fix not enough arguments for format string
The most straightforward solution to this error is to provide the correct number of arguments for the format string. This means ensuring you’re providing enough arguments to fill all the placeholders in the string and not providing too many arguments that don’t correspond to any placeholders.
Here is an example of string formatting with the correct number of arguments:

Another problem-solving approach to this issue is incorporating f-strings. Formatted string literals, or “fstrings”, supply us with a means of interweaving expressions inside our text strings by using curly braces {}. They were introduced in Python 3.6 and have since become a popular way to format strings.
To use fstrings, you add an f before the opening quotation mark of the string literal. You can include any valid Python expression wrapped in curly braces inside the string. When the string is evaluated, the expressions are replaced with their values.
Let’s take a look at an example to understand how fstrings can be used to format strings in Python:
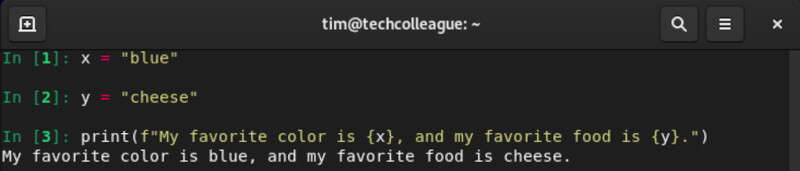
Best Practices for Avoiding the Error
First and foremost, I recommend taking a closer look at the string format syntax in your code before running it. Ensure you use the correct syntax for placeholders and do not accidentally miss the parentheses.
Another best practice is to avoid using string concatenation to construct complex strings. Instead, use string formatting or interpolation techniques like f-strings to make your code more readable and maintainable.
Finally, testing your code thoroughly before running it in a production environment is always a good idea. This can help you catch any errors or bugs before they cause problems.
By following these best practices and taking care when formatting strings in your Python code, you can avoid encountering the “TypeError: not enough arguments for format string” error altogether.
Related: TypeError: ‘tuple’ object is not callable in Python
Conclusion
The “TypeError: not enough arguments for format string” error can be a frustrating issue to deal with in your Python code. However, you can avoid encountering this error by understanding the common causes, implementing the solutions I’ve outlined, and following best practices for string formatting.
Remember to double-check the syntax of your string format and the number of arguments you’re passing. Consider using f-strings to make your code more readable, and avoid using string concatenation to construct complex strings.
By taking these steps and thoroughly testing your code, you can write clean, readable, and error-free Python code that will impress you.