
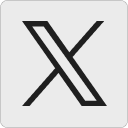




If you’re new to Python or programming in general, you might have heard the terms “List,” “Tuple,” “Set,” and “Dictionary” being thrown around. These are all examples of data structures that are essential to programming.
In Python, these data structures help us organize and manipulate our data more efficiently. Each has unique characteristics and uses, so it’s important to understand their differences to choose the right one for your task.
In this article, I’ll comprehensively overview List, Tuple, Set, and Dictionary in Python. We’ll cover their definition, creation, manipulation, and access of elements, as well as their advantages and use cases.
So, let’s get started!
List
List is one of the most commonly used data structures in Python. It’s an ordered collection of items from different data types like integers, strings, or other lists.
To create a List in Python, you enclose the items in square brackets, separated by commas.
For example, a List of fruits could be created like this:

To access elements in a List, we can use their index numbers. In Python, indexing starts from 0, so the first element of the List would have an index of 0, the second element would have an index of 1, and so on.
For example, we would use fruits to access the first fruit in our List of fruits[0]. This would output “apple” to the console.
We can also manipulate the elements of a List in various ways. For example, we can add elements to a List using the append()
method, like this:
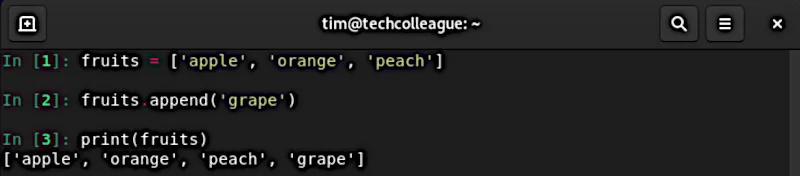
This would add “grape” to the end of our List of fruits. We can also remove elements from a List using the remove()
method, like this:
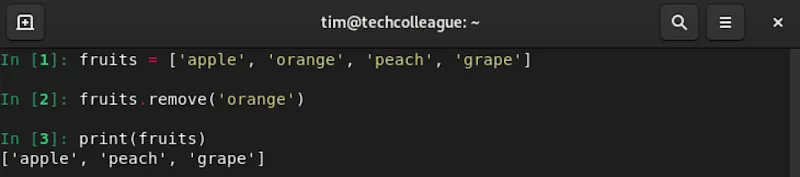
This would remove “banana” from our List of fruits.
There are many other methods and operations that we can perform on a List in Python, like sorting the elements using the sort()
method, or checking if an element exists in the List using the in
keyword.
Lists are a powerful and versatile data structure in Python that can be used in many ways.
Tuple
Tuple is another data structure in Python that is similar to List, but with a few key differences. Like List, Tuple is also an ordered collection of elements, but unlike List, Tuple is immutable, which means that once a Tuple is created, its elements cannot be changed.
To create a Tuple in Python, we enclose the elements in parentheses instead of square brackets, separated by commas.
For example, a Tuple of coordinates could be created like this:

To access elements in a Tuple, we use the same indexing as we do with Lists. For example, we would use coordinates to access the first element in our Tuple of coordinates[0]. This would output “3” to the console.
Although we cannot change the elements of a Tuple, we can still perform various operations on it. For example, we can concatenate two Tuples using the +
operator, like this:
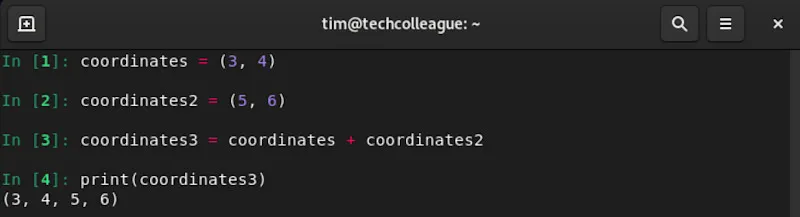
This would create a new Tuple coordinates3
with elements (3, 4, 5, 6)
.
There are also Tuple methods and operations like count()
and index()
that can be used to manipulate Tuples.
Tuples are often used to ensure the data remains unchanged throughout the program. They can also be used as keys in a Dictionary, which we’ll cover later in this article.
Tuples are a useful data structure in Python that can be used when storing a fixed set of elements that should not be changed.
Related: Python Import From Parent Directory
Set
Set is another data structure in Python that is used to store a collection of unique elements. Unlike List and Tuple, Sets are not ordered, meaning we cannot access their elements using indexing.
To create a Set in Python, we enclose the elements in curly braces, separated by commas. For example, a Set of even numbers could be created like this:

Sets can also be created from Lists using the set()
method, like this:

To add elements to a Set, we use the add()
method, like this:
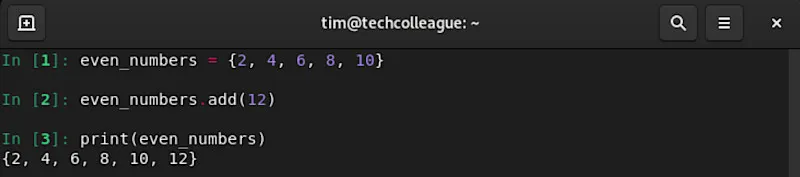
This would add “12” to our Set of even numbers. We can also remove elements from a Set using the remove()
method, like this:
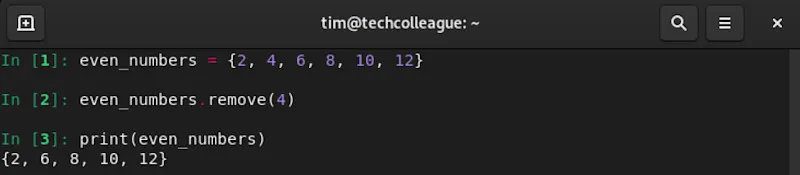
This would remove “4” from our Set of even numbers.
Sets have a few useful operations that can be used to manipulate them. For example, we can use the union()
method to combine two Sets, like this:
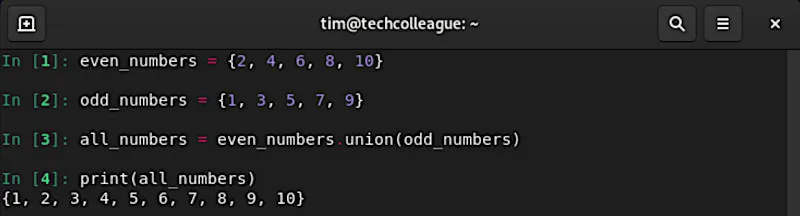
This would create a new Set all_numbers
with elements (1, 3, 4, 5, 6, 7, 8, 9, 10)
.
We can also use Set operations like intersection()
, difference()
, and symmetric_difference()
to perform various set operations on Sets.
Sets are a useful data structure in Python when we store unique elements and perform set operations.
Dictionary
Dictionary is another data structure in Python that stores a collection of key-value pairs. Unlike List, Tuple, and Set, Dictionaries are not ordered, instead, they are indexed by their keys.
To create a Dictionary in Python, we enclose the key-value pairs in curly braces, separated by colons.
For example, a Dictionary of fruits and their corresponding colors could be created like this:

To access the value of a specific key in a Dictionary, we use the key as the index. This would output “red” to the console.
We can also add new key-value pairs to a Dictionary using the following syntax:
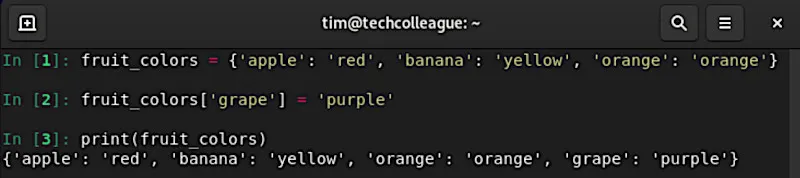
This would add a new key-value pair to our Dictionary with the key “grape” and the value “purple”.
We can also remove key-value pairs from a Dictionary using the del
keyword, like this:
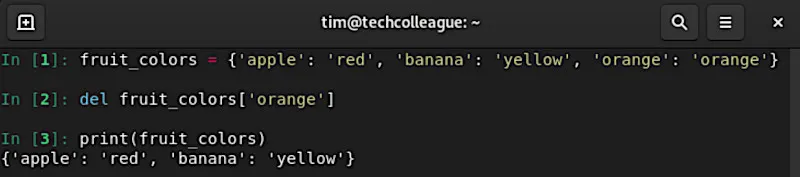
This would remove the key-value pair with the key “orange” from our Dictionary.
Dictionaries have a few useful operations that can be used to manipulate them. For example, we can use the keys()
method to get a list of all the keys in a Dictionary, like this:

This would output dict_keys(['apple', 'banana', 'orange'])
to the console.
We can also use the values()
method to get a list of all the values in a Dictionary, like this:

This would output dict_values(['red', 'yellow', 'orange'])
to the console.
Dictionaries are often used when storing data in a key-value format and quickly accessing the values using the keys. They are also useful for implementing various algorithms and data structures in Python.
Comparison of List, Tuple, Set, and Dictionary
List, Tuple, Set, and Dictionary are different data structures in Python that store collections of elements. Each data structure has strengths and weaknesses, and choosing the right one for a particular situation can make our code more efficient and easier to understand.
Lists are a very versatile data structure in Python that can store elements of any data type and be modified after creation. However, accessing elements in a List using indexing can be slow when dealing with large Lists, and checking for membership in a List can also be slow.
Conversely, Tuples are similar to Lists but immutable, meaning their elements cannot be modified after creation. This makes Tuples faster and more memory-efficient than Lists, but it also means that we cannot add or remove elements from a Tuple once it has been created.
Sets are a data structure in Python that stores collections of unique elements. They are very fast when checking for membership or performing set operations like union, intersection, and difference. However, Sets are unordered, meaning we cannot access their elements using indexing.
Dictionaries are a data structure in Python that stores key-value pairs’ collections. They are very fast when looking up values using keys, but they are unordered, meaning we cannot access their elements using indexing. Additionally, Dictionaries require more memory than Lists or Tuples because they have to store both keys and values.
We should choose the appropriate data structure based on the specific requirements of our code. Sets are the way to go if we need to store a collection of unique elements and perform set operations on them.
Dictionaries are the best option to store a collection of key-value pairs and quickly look up values using keys. Lists and tuples are more general-purpose data structures that can be used in various situations, depending on the specific needs of our code.
Conclusion
Understanding the differences between List, Tuple, Set, and Dictionary is an important part of becoming proficient in Python programming. Each data structure has unique characteristics, and choosing the right one for a particular task can make our code more efficient and easier to understand.
Lists and tuples are great for storing collections of elements, with Tuples being faster and more memory-efficient than Lists. Sets are ideal for storing unique elements and performing set operations, while Dictionaries are great for storing collections of key-value pairs and quickly looking up values using keys.
As we continue to develop our Python programming skills, we will encounter many situations where we need to use one or more of these data structures to solve a particular problem. By understanding the strengths and weaknesses of each data structure, we can choose the right one for the task at hand and write more efficient and effective code.
I hope this article has helped you better understand List, Tuple, Set, and Dictionary in Python.
Happy coding!