
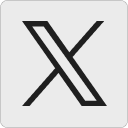




If you’re reading this, chances are you’ve encountered a frustrating error message while trying to run a Python script: “Errno 13 Permission Denied.” This error occurs when you don’t have the necessary permissions to access a file or directory.
In this article, I’ll show you how to fix this error and get your script up and running again.
What Causes the “Errno 13 Permission Denied” Error?
Before diving into the solutions, let’s first understand what causes this error. The Python “Errno 13 Permission Denied” error is raised when you try to access a file or directory because you don’t have the necessary permissions.
Here are a few common scenarios where you might encounter this error:
- You are trying to write to a file owned by another user, and you don’t have the necessary permissions to do so.
- You are trying to write to a file you own, but its permissions don’t allow you to write to it.
- You are trying to execute a script owned by another user and don’t have the necessary permissions.
Here is an example of the [Errno 13] Permission Denied error:
![Python [Errno 13] Permission Denied](https://techcolleague.com/wp-content/uploads/2023/01/python-errno-13-permission-denied.jpg)
How to Fix [Errno 13] Permission Denied in Python?
Now that we understand what causes the “Errno 13 Permission Denied” error let’s look at some ways to fix it.
Check the File or Directory Permissions
The first thing you should do when trying to fix this error is to check the permissions of the file or directory you are trying to access.
Here’s how to do that:
- Open a terminal window.
- Navigate to the directory containing the file or directory you are trying to access.
- Use the
ls -l
command to list the contents of the directory, along with their permissions.

- Look at the permissions of the file or directory in question. To indicate the file type, the first character in a permissions string is used (e.g., ‘d’ for directories and ‘-‘ for regular files). The next three characters represent the permissions for the file owner, the next three represent the permissions for the group, and the final three represent the permissions for everyone else.
- As shown above, the file is owned by
john
, but you are logged in astim
. You will need to change the permissions to fix the error.
Change the File or Directory Permissions
If the file or directory permissions don’t allow you access, you’ll need to change the permissions to fix the error.
Here’s how to do that:
- Open a terminal window.
- Navigate to the directory containing the file or directory you are trying to access.
- Use the
chmod
command to change the permissions of the file or directory. For example, if you want to give yourself read and write permissions for a file calledmedia.txt
, you would use the following command:
chmod u+rw media.txt
This command gives the owner (u
) read and write (rw
) permissions for the file.
You can also use the chmod
command to change the permissions for the group or everyone else. For example, to give the group read and execute permissions for a directory called myfolder
, you would use the following command:
chmod g+rx myfolder
This command gives the group (g
) read and execute (rx
) permissions for the directory.
If you want to give everyone else read and execute permissions for a file, you can use the following command:
chmod o+rx media.txt
This command gives everyone else (o
) read and execute (rx
) permissions for the file.
Related: [Solved] “TypeError: ‘list’ object is not callable” in Python
Use sudo
to Run the Script with Root Permissions
If you are trying to execute a script owned by another user, you may need to use the sudo command to run the script with root permissions. The sudo command allows you to execute a command as the root user or as another user with the necessary permissions.
Here’s how to use the sudo
command to run a script:
- Open a terminal window.
- Navigate to the directory containing the script you want to run.
- Use the
sudo
command to run the script. For example, to run a script calledmyscript.py
, you would use the following command:
sudo python myscript.py
This command will prompt you for your password and then run the script with root permissions.
Remember that using the sudo command can be dangerous, as it allows you to execute commands with root permissions. Use it with caution and only when necessary.
Use os.chmod()
to Change the Permissions of the File or Directory
If you are trying to access a file or directory from within a Python script, you can use the os
module’s chmod()
function to change the permissions of the file or directory.
Here’s how to use the os.chmod()
function:
- Import the
os
module at the top of your Python script.
import os
- Use the
os.chmod()
function to change the permissions of the file or directory. For example, to give yourself read and write permissions for a file calledmedia.txt
, you would use the following code:
os.chmod("media.txt", 0o600)
This code gives the owner read and write permissions for the file (0o600
is an octal representation of the permissions, with 6
representing read and write permissions).
You can also use the os.chmod()
function to change the permissions for the group or for everyone else. For example, to give the group read and execute permissions for a directory called mydir
, you would use the following code:
os.chmod("mydir", 0o040)
This code gives the group read and execute permissions for the directory (0o040
is an octal representation of the permissions, with 4
representing read and 0
representing execute permissions).
If you want to give everyone else read and execute permissions for a file, you can use the following code:
os.chmod("media.txt", 0o004)
This code gives everyone else read and execute permissions for the file (0o004
is an octal representation of the permissions, with 4
representing read and 0
representing execute permissions).
Use os.umask()
to Change the Default Permissions for New Files and Directories
If you find that you are frequently encountering the “Errno 13 Permission Denied” error when trying to access new files or directories that you create, you can use the os
module’s umask()
function to change the default permissions for new files and directories.
Here’s how to use the os.umask()
function:
- Import the
os
module at the top of your Python script.
import os
- Use the
os.umask()
function to change the default permissions for new files and directories. For example, to set the default permissions for new files and directories to0o755
, you would use the following code:
os.umask(0o022)
This code sets the default permissions for new files and directories to 0o755
(0o755
is an octal representation of the permissions, 7
representing read, write, and execute permissions).
Keep in mind that the umask()
function only affects the permissions of new files and directories created after the function is called. It does not affect the permissions of existing files and directories.
Conclusion
I hope this article has helped you understand what causes the “Errno 13 Permission Denied” error and how to fix it.
If you require to view and modify the permissions of a file or dir, utilize the sudo command for executing scripts with root privileges, or call on the os.chmod() or os.umask() functions from inside Python script, then look no further.
You now have all that is necessary to diagnose and fix this error quickly and effectively. Good luck, and happy coding!