
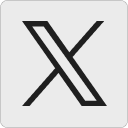




The “while” loop is a fundamental building block in bash scripting, allowing you to execute commands repeatedly until a certain condition is met. In this article, we will take a deep dive into the usage of while loops in bash, providing detailed examples and explanations to help you master this powerful tool.
We’ll start by introducing the basic syntax of a while loop, then move on to more advanced examples, covering topics such as counting, reading from files, and user input. We’ll also take a look at some frequently asked questions to help you troubleshoot any issues you may encounter along the way.
Whether you’re a beginner or an experienced bash scripter, this article has something for everyone.
Let’s get started!
Bash While Loop Examples
A while loop in Bash executes a set of commands repeatedly until a certain condition is met. The basic syntax of a while loop is as follows:
while [condition]; do
commands
done
The condition is evaluated before each iteration of the loop, and if it is true, the commands within the loop are executed. Once the condition is false, the loop will exit.
Here are some examples of using the while loop in bash:
Example 1: Counting to 10
# Initialize the counter variable
counter=1
# Set the condition for the loop to continue
while [ $counter -le 10 ]; do
echo $counter
((counter++))
done
In this example, we initialize a variable called “counter” with a value of 1. We then set the condition for the loop to continue as long as the value of “counter” is less than or equal to 10.
Within the loop, we use the “echo” command to print the current value of the counter and then increment the counter by 1 using the double parenthesis notation. This loop will run 10 times, printing the numbers 1 through 10.
Example 2: Reading from a File
# Set the file to read from
file="data.txt"
# Set the condition for the loop to continue
while read line; do
echo $line
done < $file
In this example, we set a variable called “file” to the name of a file we want to read from. We then set the condition for the loop to continue as long as there is a line to read from the file.
We use the “echo” command within the loop to print the current line from the file. This loop will run until there are no more lines to read from the file.
Related: Bash Concatenate Strings
Example 3: User Input
# Set the condition for the loop to continue
while true; do
read -p "Enter a number: " num
if [[ $num =~ ^[0-9]+$ ]]; then
echo "You entered the number: $num"
break
else
echo "Invalid input. Please enter a number."
fi
done
In this example, we set the condition for the loop to continue as true, which means the loop will run indefinitely until a “break” command is encountered. Within the loop, we use the “read” command to read input from the user and store it in a variable called “num.”
We then use an if statement and regular expression to check if the user’s input is a valid number. If it is, we print the number and break out of the loop. If it is not, we print an error message and the loop continues.
Conclusion
While loops are a powerful tool in bash scripting, allowing you to execute commands repeatedly until a certain condition is met. By understanding the basic syntax and usage of while loops, you can use them to accomplish many different tasks.
Remember always to be mindful of the loop’s exit condition, as an infinite loop can cause unexpected behavior.
Frequently Asked Questions
How do I check the value of a variable within the while loop condition?
You can check the value of a variable within the while loop condition by using the double square bracket notation, like so:
while [[ condition ]]; do
commands
done
For example, if you want to check if the value of a variable “counter” is less than or equal to 10, the condition would be:
[[ $counter -le 10 ]]
How do I increment a variable within the while loop?
You can increment a variable within the while loop by using the double parenthesis notation, like so:
((variable++))
For example, if you want to increment the value of the “counter” variable by 1, you would use the following command:
((counter++))
How do I break out of a while loop?
You can break out of a while loop by using the “break” command. This will immediately exit the loop and continue the execution of any commands that come after the loop. For example:
while [[ condition ]]; do
commands
if [[ some_condition ]]; then
break
fi
done
In this example, if the “some_condition” is true, the loop will exit, and the commands after the loop will continue to execute.
How do I continue the next iteration of a while loop?
You can continue the next iteration of a while loop by using the “continue” command. This will immediately jump to the next loop iteration, skipping any commands after the continue statement. For example:
while [[ condition ]]; do
if [[ some_condition ]]; then
continue
fi
commands
done
In this example, if the “some_condition” is true, the commands after the continue statement will be skipped, and the loop will continue to the next iteration.