
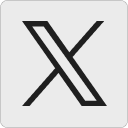




As a Python developer, you may have encountered the “KeyError: 0” exception at some point in your career. This error occurs when you try to access a key in a dictionary or set, but the key does not exist.
In this article, we’ll look closely at the “KeyError: 0” exception and how to fix it.
What Causes the KeyError: 0 Exception?
The “KeyError: 0” exception is caused when you try to access a key in a dictionary or set, but the key does not exist.
For example, consider the following code:
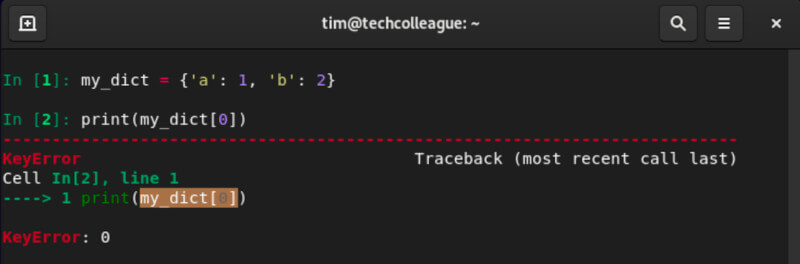
In this code, we are trying to access the key 0
in the dictionary my_dict
. However, 0
is not a key in the dictionary, so Python raises a “KeyError: 0” exception.
How to Fix KeyError: 0 Exception in Python?
There are a few different ways to fix the “KeyError: 0” exception, depending on the specific context of your code.
Here are a few options:
Option 1: Check if the Key Exists
The most straightforward way to fix the “KeyError: 0” exception is to check if the key you are trying to access exists in the dictionary or set.
For example:
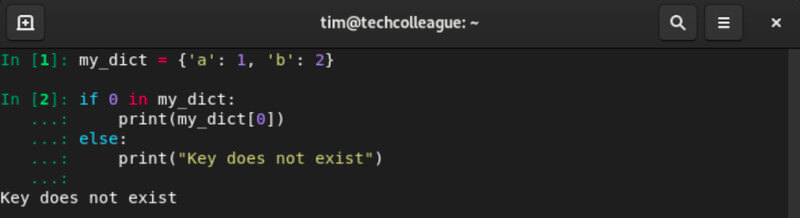
In this code, we use the in
operator to check if the key 0
exists in the dictionary my_dict
. If it does, we access the value associated with that key. If it does not, we print a message indicating that the key does not exist.
Related: How to Fix Command “python setup.py egg_info” failed with error code 1
Option 2: Use a Default Value
Another option for fixing the “KeyError: 0” exception is to use a default value when accessing a key in a dictionary.
For example:

In this code, we use the dict.get()
method to try to access the key 0
in the dictionary my_dict
. If the key does not exist, the get()
method returns the default value "Key does not exist"
instead of raising an exception.
Option 3: Use a Defaultdict
A third option for fixing the “KeyError: 0” exception is to use a defaultdict
from the collections
module. A defaultdict
is a subclass of the built-in dict
class that allows you to specify a default value for keys that do not exist.
For example:
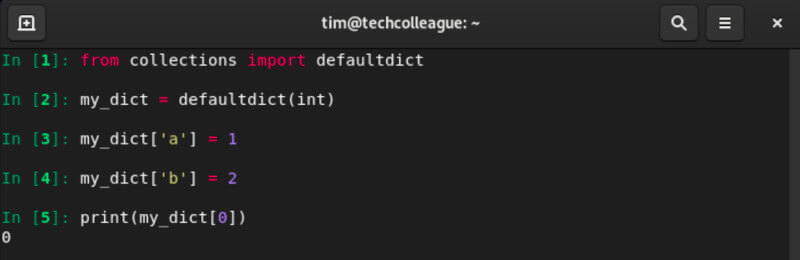
In this code, we create a defaultdict
with a default value of int
, which means that any keys that do not exist will have a default value of 0
. When we try to access the key 0
, the defaultdict
returns the default value 0
instead of raising a “KeyError: 0” exception.
Other Tips for Debugging Key Errors
In addition to the strategies for fixing the “KeyError: 0” exception, here are a few other tips for debugging key errors in Python:
Use the dir()
Function to Explore Objects
If unsure of the keys or attributes available on an object, you can use the dir()
function to explore its contents.
For example:
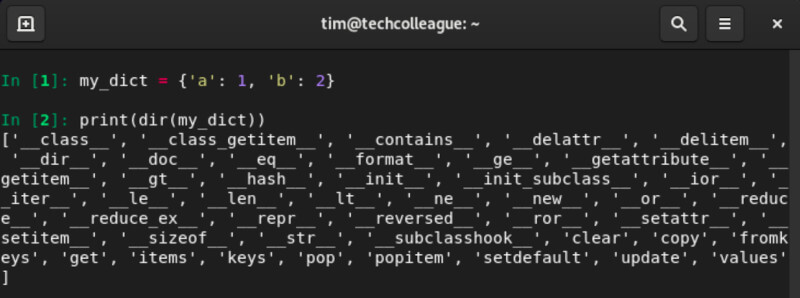
This code will print a list of all the keys, methods, and attributes available on the my_dict
object.
Use the pprint()
Function to Explore Data Structures
If you are working with a large or complex data structure, you may find it helpful to use the pprint()
function from the pprint
module to print it in a more readable format.
For example:
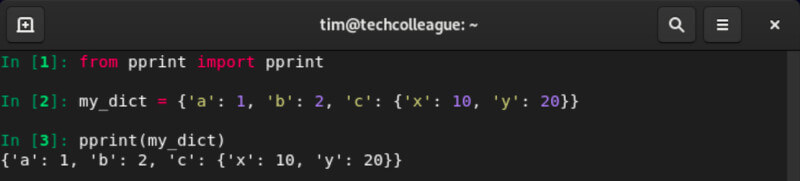
This code will print the my_dict
object in a more readable, indented format.
Conclusion
This article looked at Python’s “KeyError: 0” exception and how to fix it. We explored a few different strategies for handling key errors, including checking if the key exists, using a default value, and using a defaultdict
.
We also covered some tips for debugging key errors, such as using the dir()
function to explore objects, and the pprint()
function to print complex data structures in a more readable format.
By understanding the causes and solutions for the “KeyError: 0” exception, you’ll be better equipped to troubleshoot and fix key errors in your Python code. As you continue developing your Python skills, it’s important to remember the various ways exceptions can occur and how to handle them effectively.